PHP Exercises: Decapitalize the first letter of the string and then adds it with rest of the string
PHP: Exercise-97 with Solution
Write a PHP program to decapitalize the first letter of the string and then adds it with rest of the string.
Sample Solution:
PHP Code:
<?php
// Licence: https://bit.ly/2CFA5XY
// Function definition for 'decapitalize' that takes a string and an optional parameter '$upperRest' (default is false)
function decapitalize($string, $upperRest = false)
{
// Use 'lcfirst' to convert the first character of the string to lowercase
// Use 'strtoupper' if '$upperRest' is true, otherwise keep the string as it is
return lcfirst($upperRest ? strtoupper($string) : $string);
}
// Call 'decapitalize' with a string and display the result using 'print_r'
print_r(decapitalize('Python'));
?>
Sample Output:
python
Flowchart:
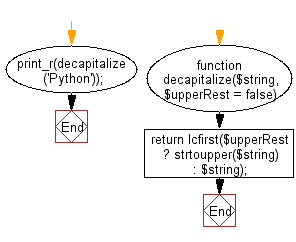
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a PHP program to count number of vowels in a given string.
Next: Write a PHP program to create a new function that composes multiple functions into a single callable.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics