PHP Exercises: Count number of vowels in a given string
PHP: Exercise-96 with Solution
Write a PHP program to count number of vowels in a given string.
Note: Use a regular expression to count the number of vowels (A, E, I, O, U) in a string.
Sample Solution:
PHP Code:
<?php
// Licence: https://bit.ly/2CFA5XY
// Function definition for 'count_Vowels' that takes a string as a parameter
function count_Vowels($string)
{
// Use 'preg_match_all' with a regular expression to find all vowels (case-insensitive) in the string
preg_match_all('/[aeiou]/i', $string, $matches);
// Return the count of matched vowels found in the string
return count($matches[0]);
}
// Call 'count_Vowels' with a string and display the result using 'print_r'
print_r(count_Vowels('sampleInput'));
?>
Sample Output:
4
Flowchart:
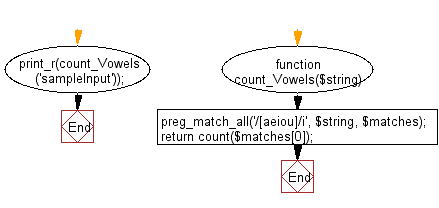
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a PHP program to check if a given string starts with a given substring.
Next: Write a PHP program to decapitalize the first letter of the string and then adds it with rest of the string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics