PHP Exercises: Filter out the elements of a given array, that have one of the specified values
92. Filter Array to Exclude Specified Values
Write a PHP program to filter out the elements of a given array, that have one of the specified values.
Sample Solution:
PHP Code:
<?php
// Licence: https://bit.ly/2CFA5XY
// Function definition for 'without' that takes an array reference and a variable number of parameters as input
function without($items, ...$params)
{
// Use 'array_diff' to remove elements specified by the parameters from the array
// Use 'array_values' to reindex the array after removal
return array_values(array_diff($items, $params));
}
// Call 'without' with an array and specified values to be removed, then display the result using 'print_r'
print_r(without([2, 1, 2, 3], 1, 2));
?>
Explanation:
- Function Definition:
- The function without is defined to accept an array reference ($items) and a variable number of parameters (...$params).
- The ...$params syntax allows the function to accept multiple arguments after the first array parameter.
- Removing Specified Elements:
- Inside the function, array_diff($items, $params) is used:
- This function compares the $items array with the values in $params and returns a new array containing only the values from $items that are not in $params.
- Reindexing the Array:
- The result of array_diff is passed to array_values(), which reindexes the resulting array, ensuring that the keys are sequential integers starting from 0.
- Purpose:
- The without function effectively creates a new array that excludes any elements specified in the parameters.
- Function Call:
- The function is called with an array [2, 1, 2, 3] and the values 1 and 2 to be removed.
- The result of this call is print_r(without([2, 1, 2, 3], 1, 2));, which outputs [3], as 1 and 2 are removed from the original array.
- Displaying Results:
- The final result, which is the array [3], is displayed using print_r.
Output:
Array ( [0] => 3 )
Flowchart:
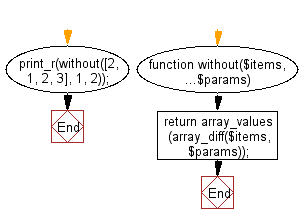
For more Practice: Solve these Related Problems:
- Write a PHP script to iterate over an array and filter out elements that are in a given exclusion list.
- Write a PHP function to return a new array containing only the elements not matching any value in a specified blacklist.
- Write a PHP script to use a custom callback to remove unwanted items from an array and display the result.
- Write a PHP script to compare each array element against a set of forbidden values and output the filtered array.
Go to:
PREV : Remove n Elements from the Beginning of an Array.
NEXT : Sort Collection by a Specific Key.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.