PHP Exercises: Changes the color of first character of a word
9. Change Color of First Character
Write a PHP script, which changes the color of the first character of a word.
Sample string : PHP Tutorial
Sample Solution:
PHP Code:
<?php
// Define a text string
$text = 'PHP Tutorial';
// Use a regular expression to replace the first letter of each word with a span element with red color
$text = preg_replace('/(\b[a-z])/i', '\1', $text);
// Display the modified text with styled first letters
echo $text;
?>
Sample Output:
PHP Tutorial
View the output in the browser
Explanation:
Here's a brief explanation of the above exercise:
- $text = 'PHP Tutorial';
- Defines a text string with the content "PHP Tutorial."
- $text = preg_replace('/(\b[a-z])/i', '<span style="color:red;">\1</span>', $text);
- Uses a regular expression (/(\b[a-z])/i) with preg_replace() to replace the first letter of each word in the text with a <span> element having red color style. The regular expression looks for word boundaries (\b) followed by any lowercase letter ([a-z]). The 'i' modifier makes the matching case-insensitive.
- echo $text;
- Displays the modified text, where the first letter of each word has been replaced with a styled <span> element in red color.
preg_replace() function:
Syntax:
mixed preg_replace ( mixed $pattern , mixed $replacement , mixed $subject [, int $limit = -1 [, int &$count ]] )
Searches subject for matches to pattern and replaces them with replacement. The function returns an array if the subject parameter is an array, or a string otherwise. If matches are found, the new subject will be returned, otherwise subject will be returned unchanged or NULL if an error occurred.
Flowchart:
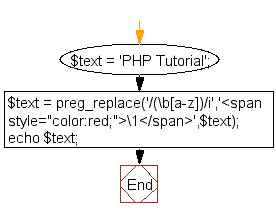
For more Practice: Solve these Related Problems:
- Write a PHP script to wrap the first character of every word in a string with a span tag for custom styling.
- Write a PHP script to apply a random color to the first character of a list of words in a sentence.
- Write a PHP script to change the font color of the first character in multiple sentences of a paragraph.
- Write a PHP script to create a function that takes any string and returns it with a styled first letter.
Go to:
PREV : Parse URL Components.
NEXT : Detect Page Protocol.
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.