PHP Exercises: Filter the collection using the given callback
Write a PHP program to filter the collection using the given callback.
Sample Solution:
PHP Code:
<?php
// Licence: https://bit.ly/2CFA5XY
// Function definition for 'reject' that takes an array of items and a filtering function as parameters
function reject($items, $func)
{
// Use 'array_filter' to filter the items based on the given function
// Use 'array_diff' to get the items that are not present in the filtered array
// Use 'array_values' to reindex the resulting array
return array_values(array_diff($items, array_filter($items, $func)));
}
// Call 'reject' with an array and an anonymous function filtering items with a length greater than 4, then display the result using 'print_r'
print_r(reject(['Green', 'Red', 'Black', 'White'], function ($item) {
return strlen($item) > 4;
}));
?>
Explanation:
- Function Definition:
- The function reject is defined to take two parameters:
- $items: an array of items to be filtered.
- $func: a filtering function that determines which items to reject.
- Filtering Items:
- Inside the function:
- array_filter($items, $func) is used to create an array of items that pass the condition specified in $func.
- This array contains only the items for which the filtering function returns true.
- Getting Rejected Items:
- array_diff($items, array_filter($items, $func)) is then used to find the items in the original array ($items) that are not present in the filtered array.
- This effectively gives the items that do not satisfy the condition defined by the filtering function.
- Reindexing the Array:
- array_values(...) is applied to the result of array_diff to reindex the resulting array, ensuring that the keys are reset in the output.
- Return Statement:
- The modified array, containing only the rejected items, is returned from the function.
- Function Call:
- The reject function is called with an array of color names (['Green', 'Red', 'Black', 'White']) and an anonymous function that filters out items with a length greater than 4.
- Displaying Results:
- The result of the reject function call is displayed using print_r, showing the array of items that do not satisfy the condition (in this case, items with length 4 or less).
- Purpose:
- The reject function effectively filters out items from an array based on a specified condition, returning only those items that do not meet the condition defined by the filtering function.
Output:
Array ( [0] => Red )
Flowchart:
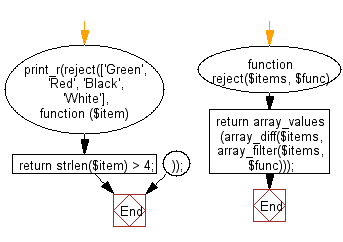
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a PHP program to mutate the original array to filter out the values specified.
Next: Write a PHP program to return all elements in an given array except for the first one.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics