PHP Exercises: Mutate the original array to filter out the values specified
88. Mutate Array to Filter Out Specified Values
Write a PHP program to mutate the original array to filter out the values specified.
Sample Solution:
PHP Code:
<?php
// Licence: https://bit.ly/2CFA5XY
// Function definition for 'pull' that takes an array reference and variable number of parameters as input
function pull(&$items, ...$params)
{
// Use 'array_diff' to remove elements specified by the parameters from the array
// Use 'array_values' to reindex the array after removal
$items = array_values(array_diff($items, $params));
// Return the modified array
return $items;
}
// Initialize an array '$items' with repeated values
$items = ['a', 'b', 'c', 'a', 'b', 'c'];
// Call 'pull' with the array and specified values to be removed, then display the result using 'print_r'
print_r(pull($items, 'a', 'c'));
?>
Explanation:
- Function Definition:
- The function pull is defined to take two parameters:
- &$items: an array passed by reference, allowing modifications directly to the original array.
- ...$params: a variable number of additional parameters representing the values to be removed from the array.
- Removing Elements:
- Inside the function:
- array_diff($items, $params) is used to create a new array that contains all elements of $items except those that match any of the values specified in $params.
- array_values() is applied to reindex the resulting array after the removal of specified elements, ensuring that the keys are reset.
- Updating the Original Array:
- The modified array (after removal of specified elements) is assigned back to $items, effectively updating the original array.
- Return Statement:
- The modified array is returned from the function.
- Example Initialization:
- An array $items is initialized with repeated values: ['a', 'b', 'c', 'a', 'b', 'c'].
- Function Call:
- The pull function is called with $items and the values 'a' and 'c' specified for removal.
- Displaying Results:
- The result of the pull function call is displayed using print_r, showing the updated array after removing the specified values.
- Purpose:
- The pull function effectively filters out specified values from an array, modifying the original array in place and returning the updated array.
Output:
Array ( [0] => b [1] => b )
Flowchart:
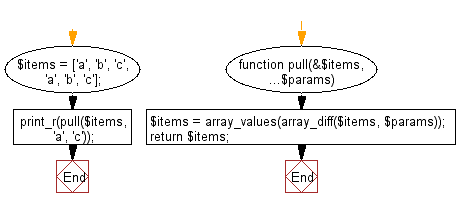
For more Practice: Solve these Related Problems:
- Write a PHP script to remove specified values from an array in place without using built-in filtering functions.
- Write a PHP function to iterate through an array and unset elements that match a provided blacklist.
- Write a PHP script to modify an original array by filtering out unwanted values using a custom loop.
- Write a PHP script to mutate an array to eliminate all occurrences of certain specified items.
Go to:
PREV : Retrieve All Values for a Given Key.
NEXT : Filter Collection Using a Callback Function.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.