PHP Exercises: Retrieve all of the values for a given key
87. Retrieve All Values for a Given Key
Write a PHP program to retrieve all of the values for a given key.
Sample Solution:
PHP Code:
Explanation:
- Function Definition:
- The function pluck is defined to take two parameters:
- $items: an array of items (either associative arrays or objects).
- $key: a string representing the key or property to extract from each item.
- Using array_map:
- Inside the function, array_map is utilized to iterate over each element in the $items array.
- A callback function is defined within array_map.
- Extracting Values:
- The callback function checks if the current item is an object:
- If it is an object, it accesses the property specified by $key (e.g., $item->$key).
- If it is an array, it accesses the array element specified by $key (e.g., $item[$key]).
- Return Statement:
- The modified array containing only the values associated with the specified key is returned.
- Example Usage:
- The pluck function is called with an array of associative arrays and the key 'name':
- It extracts the names of the products from the input array.
- The result is displayed using print_r.
- Purpose:
- The pluck function is useful for extracting a specific property or value from an array of objects or associative arrays, effectively creating a new array containing only those values.
Output:
Array ( [0] => Computer [1] => Laptop )
Flowchart:
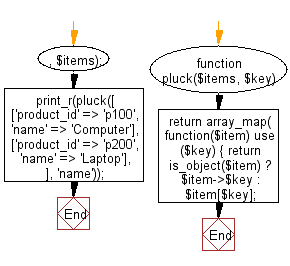
For more Practice: Solve these Related Problems:
- Write a PHP script to traverse an array of associative arrays and return all values for a specified key.
- Write a PHP function to extract column values from an array of records based on a given key.
- Write a PHP script to compile and output a list of all entries that share a common key from a multidimensional array.
- Write a PHP script to filter an array and retrieve all values associated with a target key using array_column.
Go to:
PREV : Retrieve the Last Element of a List.
NEXT : Mutate Array to Filter Out Specified Values.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.