PHP Exercises: Get the head of a given list
Write a PHP program to get the head of a given list.
Sample Solution:
PHP Code:
Explanation:
- Function Definition:
- The function head is defined to take a single parameter, $items, which is expected to be an array.
- Retrieve First Element:
- Inside the function, reset($items) is used to reset the internal pointer of the array to its first element and return that element.
- Example Usage:
- The function is called with the array [1, 2, 3]:
- The result (first element 1) is displayed using print_r.
- A newline is printed for formatting.
- Second Example Usage:
- The function is called again with another array [2, 1, 3, -4, 5, 1, 2]:
- The result (first element 2) is displayed using print_r.
- Purpose:
- The head function serves to extract and return the first element of any given array, providing a simple way to access the initial value.
Output:
1 2
Flowchart:
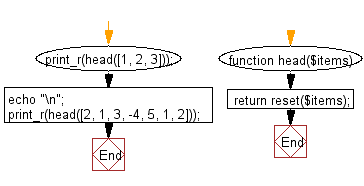
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a PHP program to check a flat list for duplicate values. Returns true if duplicate values exists and false if values are all unique.
Next: Write a PHP program to get the last element of a given list.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics