PHP Exercises: Check a flat list for duplicate values
Write a PHP program to check a flat list for duplicate values. Returns true if duplicate values exists and false if values are all unique.
Sample Solution:
PHP Code:
<?php
// Function definition for 'has_Duplicates' that takes an array of items as a parameter
function has_Duplicates($items)
{
// Check if the count of items is greater than the count of unique items
if (count($items) > count(array_unique($items)))
// If duplicates are found, return 1 (true)
return 1;
else
// If no duplicates are found, return 0 (false)
return 0;
}
// Call 'has_Duplicates' with an array containing duplicates and display the result using 'print_r'
print_r(has_Duplicates([1, 2, 3, 4, 5, 5]));
// Display a newline
echo "\n";
// Call 'has_Duplicates' with an array without duplicates and display the result using 'print_r'
print_r(has_Duplicates([1, 2, 3, 4, 5]));
?>
Explanation:
- Function Definition:
- The function has_Duplicates takes a single parameter, $items, which is expected to be an array.
- Count Comparison:
- The function checks if the total count of items in the array (count($items)) is greater than the count of unique items in the array (count(array_unique($items))).
- Return Value:
- If the count of items is greater than the count of unique items:
- The function returns 1 (indicating that duplicates are present).
- If the count of items is equal to the count of unique items:
- The function returns 0 (indicating that no duplicates are present).
- Example Usage with Duplicates:
- The function is called with an array containing duplicates: [1, 2, 3, 4, 5, 5].
- The result (1) is displayed using print_r, indicating that duplicates are found.
- Example Usage without Duplicates:
- The function is called with an array without duplicates: [1, 2, 3, 4, 5].
- The result (0) is displayed using print_r, indicating that no duplicates are found.
- Purpose:
- The has_Duplicates function provides a simple way to check for duplicate values in an array and returns a boolean-like result (1 for true, 0 for false) based on the presence of duplicates.
Output:
1 0
Flowchart:
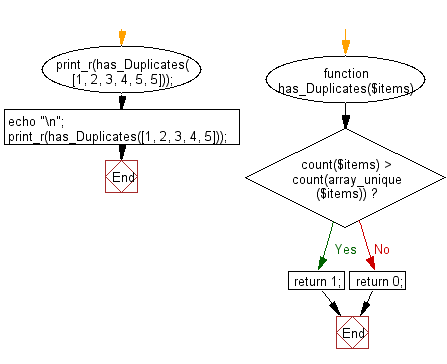
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a PHP program to group the elements of an array based on the given function.
Next: Write a PHP program to get the head of a given list.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics