PHP Exercises: Group the elements of an array based on the given function
Write a PHP program to group the elements of an array based on the given function.
Sample Solution:
PHP Code:
<?php
// License: https://bit.ly/2CFA5XY
// Function definition for 'groupBy' that takes an array of items and a grouping function as parameters
function groupBy($items, $func)
{
// Initialize an empty associative array to store the grouped items
$group = [];
// Iterate through each item in the array
foreach ($items as $item) {
// Check if the grouping function is a callable function or a valid function name
if ((!is_string($func) && is_callable($func)) || function_exists($func)) {
// If callable, use 'call_user_func' to get the key and group the item
$key = call_user_func($func, $item);
$group[$key][] = $item;
}
// Check if the item is an object
elseif (is_object($item)) {
// If an object, use the property specified by the grouping function as the key and group the item
$group[$item->{$func}][] = $item;
}
// Check if the item has a key specified by the grouping function
elseif (isset($item[$func])) {
// If set, use the specified key as the grouping key and group the item
$group[$item[$func]][] = $item;
}
}
// Return the associative array containing the grouped items
return $group;
}
// Call 'groupBy' with an array of strings and a grouping function based on string length, then display the result using 'print_r'
print_r(groupBy(['one', 'two', 'three', 'four'], 'strlen'));
?>
Explanation:
- Define groupBy Function:
- The groupBy function takes two parameters:
- $items: an array of items to group.
- $func: a function or property name used to determine the grouping criteria.
- Initialize Grouping Structure:
- An empty associative array $group is created to store the grouped items.
- Iterate Through Items:
- A foreach loop iterates over each item in the $items array.
- Check Grouping Function:
- Callable Function:
- If $func is a callable function, call_user_func($func, $item) is used to invoke it and get the key for grouping.
- The item is added to $group under that key.
- Object Property:
- If the item is an object, it checks if $func corresponds to a property of the object and groups the item by that property.
- Array Key:
- If the item is an associative array and the key specified by $func exists, it uses that key to group the item.
- Return Grouped Items:
- After processing all items, the function returns the $group associative array containing the grouped results.
- Example Usage:
- The function is called with an array of strings and the strlen function to group the strings by their length.
- The result is displayed using print_r, showing how the strings are grouped based on their lengths (e.g., all strings with length 3 will be in one group).
- Purpose:
- The groupBy function provides a flexible way to organize and categorize items in an array based on custom criteria, whether through a function, object property, or array key.
Output:
Array ( [3] => Array ( [0] => one [1] => two ) [5] => Array ( [0] => three ) [4] => Array ( [0] => four ) )
Flowchart:
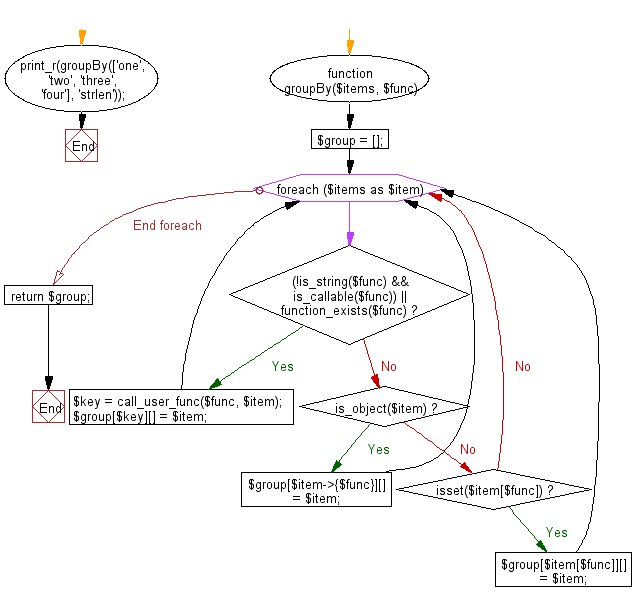
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a PHP program to get the index of the last element for which the given function returns a truth value.
Next: Write a PHP program to check a flat list for duplicate values. Returns true if duplicate values exists and false if values are all unique.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics