PHP Exercises: Get the last element for which the given function returns a truth value
81. Get Last Element Matching a Condition
Write a PHP program to get the last element for which the given function returns a truth value.
Sample Solution:
PHP Code:
<?php
// Function definition for 'find_Last' that takes an array of items and a filtering function as parameters
function find_Last($items, $func)
{
// Use 'array_filter' to filter the items based on the given function
$filteredItems = array_filter($items, $func);
// Use 'array_pop' to retrieve and return the last element from the filtered array
return array_pop($filteredItems);
}
// Call 'find_Last' with an array and an anonymous function checking for odd numbers, then display the result using 'echo'
echo find_Last([1, 2, 3, 4], function ($n) {
return ($n % 2) === 1;
});
// Display a newline
echo "\n";
// Call 'find_Last' with an array and an anonymous function checking for even numbers, then display the result using 'echo'
echo find_Last([1, 2, 3, 4], function ($n) {
return ($n % 2) === 0;
});
?>
Explanation:
- Define find_Last Function:
- The function find_Last accepts:
- $items: an array of items to search through.
- $func: a custom function used to filter the items.
- Filter Array Using Custom Function:
- array_filter($items, $func): Filters $items according to $func, resulting in an array of items that meet the function’s condition (e.g., odd or even numbers).
- Return the Last Matching Item:
- array_pop($filteredItems): Retrieves and returns the last element in filteredItems, which is the last item in $items that met the condition specified by $func.
- Function Calls and Output:
- find_Last([1, 2, 3, 4], function ($n) { return ($n % 2) === 1; }):
- Filters for odd numbers, returns 3, the last odd number.
- find_Last([1, 2, 3, 4], function ($n) { return ($n % 2) === 0; }):
- Filters for even numbers, returns 4, the last even number.
- Purpose:
- The function find_Last finds and returns the last element in an array that meets a specified condition, offering a flexible way to retrieve elements based on custom criteria.
Output:
3 4
Flowchart:
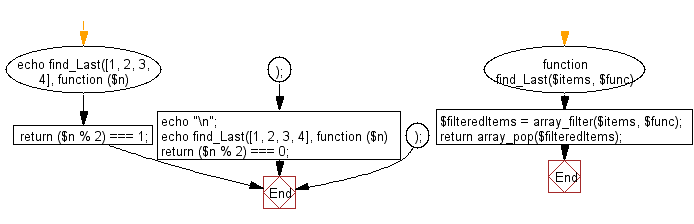
For more Practice: Solve these Related Problems:
- Write a PHP script to traverse an array from end to start and return the last element for which a given callback returns true.
- Write a PHP script to reverse iterate over a collection and output the last matching element based on a condition.
- Write a PHP function to identify the final element in an array that meets a specified criterion.
- Write a PHP script to find and return the last truthy element in an array using a custom search function.
Go to:
PREV : Remove Leftmost Elements from Array.
NEXT : Index of Last Element Matching a Condition.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.