PHP Exercises: Create a new array with n elements removed from the left
80. Remove Leftmost Elements from Array
Write a PHP program to create a new array with n elements removed from the left.
Sample Solution:
PHP Code:
<?php
// Function definition for 'drop_from_left' that takes an array of items and an optional parameter '$n' (default value is 1)
function drop_from_left($items, $n = 1)
{
// Use 'array_slice' to remove the first '$n' elements from the array
return array_slice($items, $n);
}
// Call 'drop_from_left' with an array and display the result using 'print_r'
print_r(drop_from_left([1, 2, 3]));
// Call 'drop_from_left' with an array and a specified number of elements to drop, then display the result using 'print_r'
print_r(drop_from_left([1, 2, 3, 4], 2));
?>
Explanation:
- Define drop_from_left Function:
- The function drop_from_left takes an array $items and an optional parameter $n, which defaults to 1.
- This function removes the first $n elements from the array.
- array_slice($items, $n) returns a subset of $items, starting after the first $n elements.
- If $n is not specified, the function removes only the first element (since $n defaults to 1).
- The first call, drop_from_left([1, 2, 3]), drops the first element, outputting [2, 3].
- The second call, drop_from_left([1, 2, 3, 4], 2), drops the first two elements, outputting [3, 4].
Output:
Array ( [0] => 2 [1] => 3 ) Array ( [0] => 3 [1] => 4 )
Flowchart:
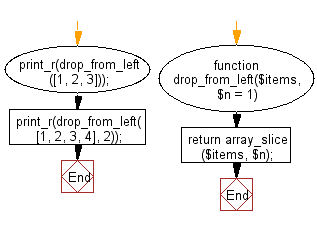
For more Practice: Solve these Related Problems:
- Write a PHP script to remove a specified number of elements from the beginning of an array and return the new array.
- Write a PHP function to create a sliced version of an array starting from a given index.
- Write a PHP script to trim n elements from the left side of an array and display the remainder.
- Write a PHP script to implement custom logic that drops the first n elements and preserves the rest.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a PHP program to deep flatten an given array.
Next: Write a PHP program to get the last element for which the given function returns a truth value.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.