PHP Exercises: Deep flatten an given array
79. Deep Array Flattening
Write a PHP program to deep flatten an given array.
Sample Solution:
PHP Code:
<?php
// Function definition for 'deep_flatten' that takes an array of items as a parameter
function deep_flatten($items)
{
// Initialize an empty array to store the flattened result
$result = [];
// Iterate through each item in the array
foreach ($items as $item) {
// Check if the current item is not an array
if (!is_array($item)) {
// If not an array, add the item to the result array
$result[] = $item;
} else {
// If the current item is an array, recursively call 'deep_flatten' on the item and merge the result with the current result array
$result = array_merge($result, deep_flatten($item));
}
}
// Return the flattened result array
return $result;
}
// Call 'deep_flatten' with a nested array as a parameter and assign the result to the variable '$result'
$result = deep_flatten([1, [2], [[3], 4], 5, 6]);
// Display the result using 'print_r'
print_r($result);
?>
Explanation:
- Define deep_flatten Function:
- The deep_flatten function takes an array, potentially containing nested arrays, and returns a single, flat array with all elements.
- Initialize an Empty Array:
- An empty array $result is created to store the flattened elements.
- Iterate Through Each Item in the Array:
- Uses a foreach loop to iterate over each item in $items.
- Check if an Item is Not an Array:
- If an item is not an array, it is appended to $result directly.
- Recursively Flatten Nested Arrays:
- If an item is an array, deep_flatten calls itself recursively on that item.
- Uses array_merge to add the flattened result of this nested array to $result.
- Return Flattened Array:
- Once all items are processed, the fully flattened array $result is returned.
- Call deep_flatten and Display Result:
- deep_flatten is called with [1, [2], [[3], 4], 5, 6], resulting in [1, 2, 3, 4, 5, 6].
- print_r outputs the final flattened array to the screen.
Output:
Array ( [0] => 1 [1] => 2 [2] => 3 [3] => 4 [4] => 5 [5] => 6 )
Flowchart:
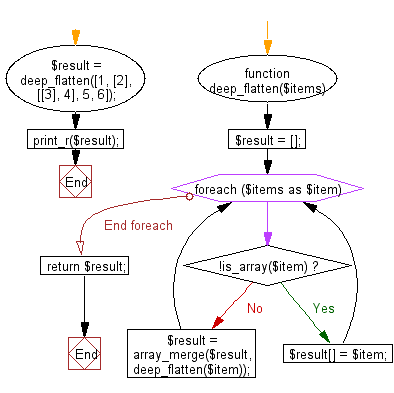
For more Practice: Solve these Related Problems:
- Write a PHP script to recursively flatten a multidimensional array into a single-level array without using built-in functions.
- Write a PHP script to traverse a nested array structure and output a flat array of all values.
- Write a PHP function to perform a deep flatten operation on an array containing nested arrays of arbitrary depth.
- Write a PHP script to iterate through an array recursively and collect all elements into a flat list.
Go to:
PREV : Array All-True Checker.
NEXT : Remove Leftmost Elements from Array.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.