PHP Exercises: Create a function that returns true for all elements of an array, false otherwise
78. Array All-True Checker
Write a PHP program to create a function that returns true for all elements of an array, false otherwise.
Sample Solution:
PHP Code:
<?php
// Function definition for 'test_all' that takes an array of items and a custom function as parameters
function test_all($items, $my_func)
{
// Check if the count of items passing the custom function is equal to the total count of items
if (count(array_filter($items, $my_func)) === count($items))
// If all items pass the condition, return 1 (true)
return 1;
else
// If at least one item fails the condition, return 0 (false)
return 0;
}
// Call 'test_all' with an array of positive numbers and a custom function checking if each item is greater than 0
echo test_all([2, 3, 4, 5], function ($item) {return $item > 0;});
// Display a newline
echo "\n";
// Call 'test_all' with an array of negative numbers and a custom function checking if each item is greater than 0
echo test_all([-2, -3, -4, -5], function ($item) {return $item > 0;});
// Display a newline
echo "\n";
// Call 'test_all' with an array of mixed positive and negative numbers and a custom function checking if each item is greater than 0
echo test_all([-2, 3, 4, -5], function ($item) {return $item > 0;});
?>
Explanation:
- Define test_all Function:
- The function test_all takes an array of items ($items) and a custom function ($my_func) as parameters.
- Check If All Items Meet a Condition:
- Uses array_filter to apply $my_func to each item in $items, creating a filtered array of items that pass the condition.
- Compares the count of items that pass the condition with the total count of $items.
- If all items pass (filtered count equals original count), returns 1 (true).
- If at least one item fails, returns 0 (false).
- Test with an Array of Positive Numbers:
- Calls test_all with [2, 3, 4, 5] and a function that checks if each item is greater than 0.
- Since all items are positive, test_all returns 1, indicating that all items pass the condition.
- Test with an Array of Negative Numbers:
- Calls test_all with [-2, -3, -4, -5] and the same condition.
- Since all items are negative, test_all returns 0, indicating that none of the items pass the condition.
- Test with a Mixed Array:
- Calls test_all with [-2, 3, 4, -5] and the same condition.
- Since not all items are positive, test_all returns 0.
Output:
1 0 0
Flowchart:
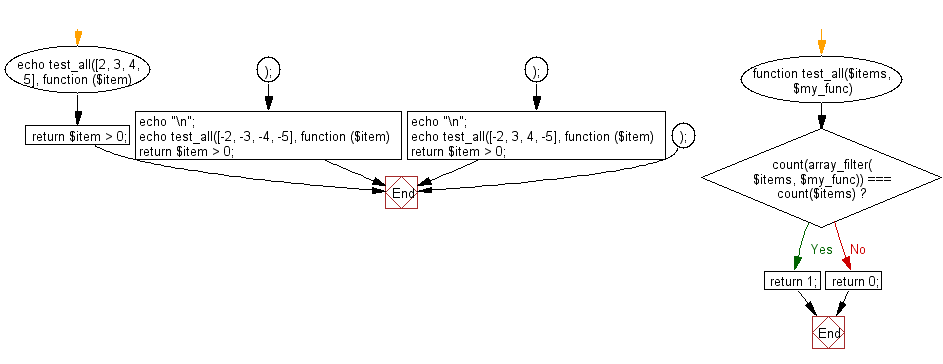
For more Practice: Solve these Related Problems:
- Write a PHP function to check if all elements in an array satisfy a given condition and return true if they do.
- Write a PHP script to iterate over an array and determine if every element evaluates as truthy.
- Write a PHP script to validate that each element in a user-defined array passes a custom boolean test.
- Write a PHP script to create a callback that returns true only if all items in an array are non-falsy values.
Go to:
PREV : Word and Page Number Indexing.
NEXT : Deep Array Flattening.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.