PHP Exercises: Reads a list of pairs of a word and a page number, and prints the word and a list of the corresponding page numbers
PHP: Exercise-77 with Solution
Write a PHP program which reads a list of pairs of a word and a page number, and prints the word and a list of the corresponding page numbers.
The number of pairs of a word and a page number is less than or equal to 1000. A word never appear in a page more than once. The words should be printed in alphabetical order and the page numbers should be printed in ascending order.
Sample Output:
The maximum value of the sum of integers passing according to the rule on one line.
Sample Solution:
PHP Code:
<?php
// Initialize an empty array to store page numbers associated with words
$page = array();
// Continuously read lines from standard input until there are no more lines
while($line = fgets(STDIN)){
// Extract word and page number from the line
list($a, $b) = explode(" ", trim($line));
// Check if the word is already in the array, if not, initialize an empty array for it
if(!isset($page[$a])){
$page[$a] = array();
}
// Add the page number to the array associated with the word
$page[$a][] = $b;
}
// Sort the array based on the keys (words)
ksort($page);
// Display a message indicating the output format
echo "The word and a list of the corresponding page numbers:\n";
// Iterate through each word and associated array of page numbers
foreach($page as $word => $arr){
// Sort the array of page numbers in ascending order
sort($arr, SORT_NUMERIC);
// Display the word followed by a newline
echo $word."\n";
// Display the sorted list of page numbers separated by spaces followed by a newline
echo implode($arr, " ")."\n";
}
?>
Sample Input:
apple 5
banana 6
Sample Output:
The word and a list of the corresponding page numbers: apple 5 banana 6
Flowchart:
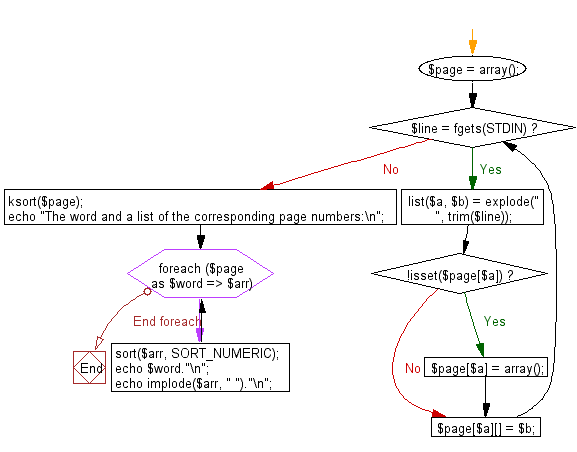
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a PHP program which adds up columns and rows of given table as shown in the specified figure
Next: Write a PHP program to create a function that returns true for all elements of an array, false otherwise.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics