PHP Exercises: Compute the maximum value of the sum of the passing integers
76. Spreadsheet Table Summation
Your task is to develop a small part of spreadsheet software.
Write a PHP program which adds up columns and rows of given table as shown in the following figure:
Pictorial Presentation:
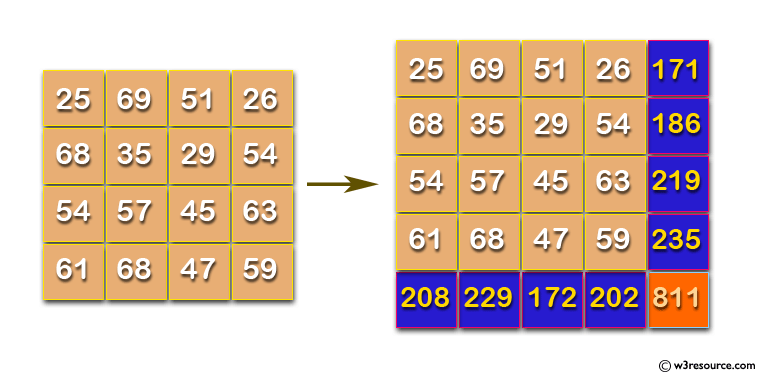
Input: n (the size of row and column of the given table)
1st row of the table
2nd row of the table
:
:
n th row of the table
The input ends with a line consisting of a single 0.
Sample Output:
The maximum value of the sum of integers passing according to the rule on one line.
Sample Solution:
PHP Code:
<?php
// Continuous loop to read input lines until '0' is entered
while (($line = trim(fgets(STDIN))) !== '0') {
// Convert the input line to an integer representing the size of the square matrix
$n = (int)$line;
// Create a 2D array (matrix) filled with zeros, with dimensions ($n + 1) x ($n + 1)
$arr = array_fill(0, $n + 1, array_fill(0, $n + 1, 0));
// Loop through each row of the matrix to populate values and calculate row and column sums
for ($i = 0; $i < $n; $i++) {
// Read a line from standard input and trim leading/trailing whitespaces
$line = trim(fgets(STDIN));
// Iterate through the elements of the current row
foreach (explode(' ', $line) as $j => $m) {
// Convert the element to an integer
$m = (int)$m;
// Assign the value to the matrix and update row and column sums
$arr[$i][$j] = $m;
$arr[$i][$n] += $m; // Update row sum
$arr[$n][$j] += $m; // Update column sum
}
}
// Calculate and assign the sum of all values in the last row and last column
$arr[$n][$n] = array_sum($arr[$n]);
// Display a message indicating the table with the sum of rows and columns
echo "The table with sum of rows and columns:\n";
// Loop through each row and column to print the matrix
for ($i = 0; $i <= $n; $i++) {
for ($j = 0; $j <= $n; $j++) {
// Format and print each matrix element
printf('%5d', $arr[$i][$j]);
}
// Move to the next line after printing a row
echo PHP_EOL;
}
}
?>
Explanation:
- Loop for Continuous Input:
- Continuously reads lines of input until the user enters '0'.
- Matrix Size Initialization:
- Converts each input line to an integer, $n, which represents the size of an n x n square matrix.
- 2D Array Initialization:
- Creates a 2D array, $arr, initialized to zero with dimensions (n+1) x (n+1).
- The extra row and column will store row and column sums.
- Matrix Population and Sum Calculation:
- Loops through each row of the matrix to populate values.
- For each row:
- Reads a line of space-separated integers and splits it into an array.
- Iterates through each integer, assigns it to the corresponding position in $arr, and updates:
- The row sum in the last column ($arr[$i][$n]).
- The column sum in the last row ($arr[$n][$j]).
- Total Sum Calculation:
- The sum of all values in the matrix is stored in the bottom-right corner ($arr[$n][$n]).
- Output the Matrix with Sums:
- Displays a message: "The table with sum of rows and columns:".
- Loops through each element in the matrix to print the matrix in a formatted way.
- Each element is printed with a width of 5, aligning values in columns.
Sample Input:
4
25 69 51 26
68 35 29 54
54 57 45 63
61 68 47 59
0
Sample Output:
The table with sum of rows and columns: 25 69 51 26 171 68 35 29 54 186 54 57 45 63 219 61 68 47 59 235 208 229 172 202 811
Flowchart:
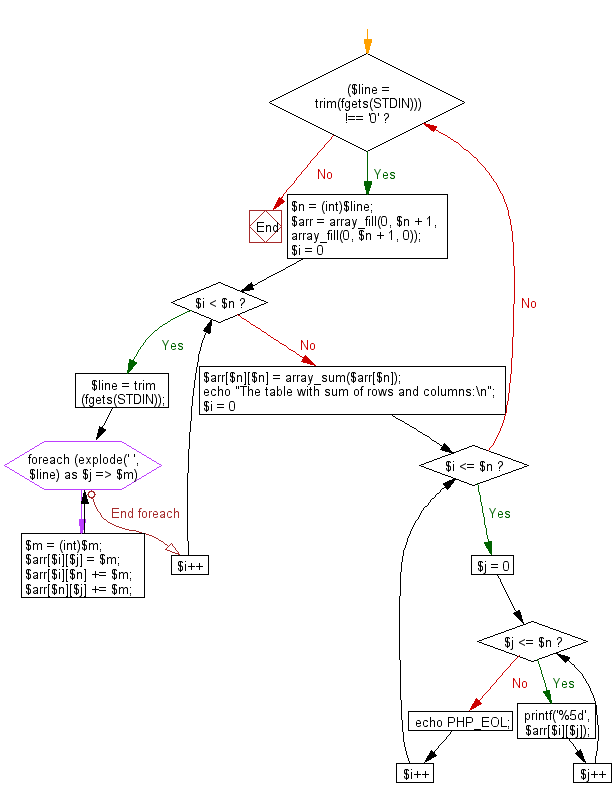
For more Practice: Solve these Related Problems:
- Write a PHP script to compute and append row and column sums to a numeric table read from input.
- Write a PHP script to generate a table with dynamically calculated margins based on row and column totals.
- Write a PHP script to process a multidimensional array and produce an augmented table showing individual and overall sums.
- Write a PHP script to validate and sum the contents of a table, then display the results in a formatted grid.
Go to:
PREV : Maximum Path Sum in a Diamond Grid.
NEXT : Word and Page Number Indexing.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.