PHP Exercises: Test whether AB and CD are orthogonal or not
68. Test Orthogonality of Lines
There are four different points on a plane, P(xp,yp), Q(xq, yq), R(xr, yr) and S(xs, ys). Write a PHP program to test whether AB and CD are orthogonal or not.
xp,yp, xq, yq, xr, yr, xs and ys are -100 to 100 respectively and each value can be up to 5 digits after the decimal point It is given as a real number including the number of.
Sample Solution:
PHP Code:
<?php
// Define a constant for a small epsilon value for numerical comparisons
define('EPS', 1e-8);
// Read input values as floating-point numbers
$a = fscanf(STDIN, '%f %f %f %f %f %f %f %f');
// Initialize an array to store the slopes of the two lines
$line = array();
// Calculate the slope of the first line, handling vertical line case
$line[] = $a[1] - $a[3] === 0.0 ? INF : ($a[0] - $a[2]) / ($a[1] - $a[3]);
// Calculate the slope of the second line, handling vertical line case
$line[] = $a[5] - $a[7] === 0.0 ? INF : ($a[4] - $a[6]) / ($a[5] - $a[7]);
// Check if the lines are orthogonal (perpendicular)
if (max($line) === INF && min($line) === 0 || abs($line[0] * $line[1] + 1.0) < EPS) {
echo 'Orthogonal';
} else {
echo 'Not orthogonal';
}
// Output a new line
echo PHP_EOL;
?>
Explanation:
- Define a Small Constant (EPS):
- EPS is set to 1e-8 to help with floating-point precision during numerical comparisons.
- Read Input Values:
- Eight floating-point numbers are read from standard input and stored in array $a. These represent the coordinates of two line segments,
- specifically:
- (x1, y1) and (x2, y2) for the first line
- (x3, y3) and (x4, y4) for the second line.
- Initialize Array for Slopes:
- An empty array $line is initialized to store the slopes of the two line segments.
- The slope of the first line is calculated as (x2 - x1) / (y2 - y1).
- If the line is vertical (y1 - y2 == 0.0), it assigns INF to indicate an undefined slope.
- Similarly, the slope of the second line is calculated as (x4 - x3) / (y4 - y3), and INF is assigned if the line is vertical.
- The code checks if the lines are orthogonal (perpendicular) by:
- Verifying if one line is vertical (INF) and the other is horizontal (0).
- Alternatively, using the formula for perpendicular slopes, where the product of the slopes should equal -1. Due to floating-point precision, abs($line[0] * $line[1] + 1.0) < EPS is used to determine if this condition is approximately true.
- Output the Result:
- If the lines are orthogonal, the output is "Orthogonal". Otherwise, it’s "Not orthogonal."
- A newline character is added at the end of the output.
Sample Input:
3.5 4.5 2.5 -1.5 3.5 1.0 0.0 4.5
Sample Output:
Not orthogonal
Flowchart:
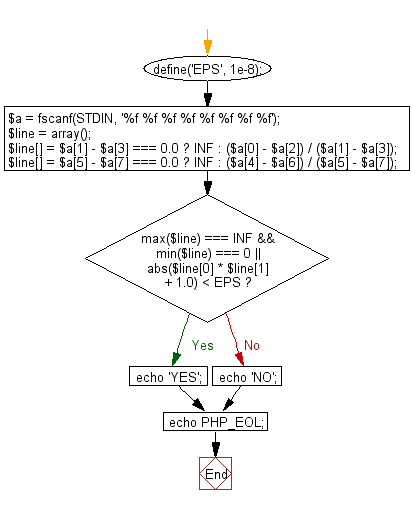
For more Practice: Solve these Related Problems:
- Write a PHP script to check if two lines, defined by two pairs of points, are orthogonal using dot product calculations.
- Write a PHP function to compute directional vectors from coordinate pairs and verify perpendicularity within a precision threshold.
- Write a PHP script to determine if two sets of points form lines that are perpendicular by checking the negative reciprocal slopes.
- Write a PHP script to validate orthogonality by computing the dot product of vectors derived from line endpoints.
Go to:
PREV : Maximum Regions with Straight Lines.
NEXT : Sum of Numerical Values in Sentence.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.