PHP Exercises: Compute the sum of first n given prime numbers
Write a PHP program to compute the sum of first n given prime numbers.
Input: n ( n ≤ 10000). Input 0 to exit the program.
Pictorial Presentation:
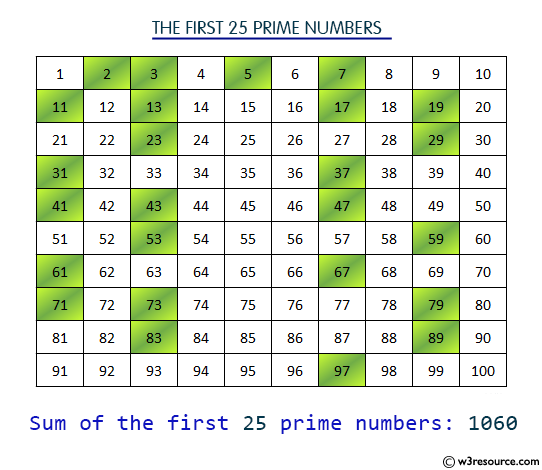
Sample Solution:
PHP Code:
<?php
// Set the maximum value for prime numbers
$max = 105000;
// Create a fixed-size array to store prime flags
$arr = new \SplFixedArray($max + 1);
// Initialize the array with 1 (considering all numbers as prime initially)
for ($i = 2; $i <= $max; $i++) {
$arr[$i] = 1;
}
// Sieve of Eratosthenes algorithm to mark non-prime numbers
for ($i = 2, $len = sqrt($max); $i <= $len; $i++) {
if (!$arr[$i]) {
continue;
}
for ($j = $i, $len2 = $max / $i; $j <= $len2; $j++) {
$arr[$i * $j] = 0;
}
}
// Process input until '0' is entered
while (($line = trim(fgets(STDIN))) !== '0') {
// Convert the input to an integer
$n = (int)$line;
// Initialize variables for result and count
$result = 0;
$cnt = 0;
// Find and sum the first N prime numbers
for ($i = 2; $i <= $max; $i++) {
if ($cnt === $n) {
break;
} elseif ($arr[$i]) {
$result += $i;
$cnt++;
}
}
// Output the result
echo "Sum of first " . $n . " prime numbers:";
echo $result, PHP_EOL;
}
?>
Explanation:
- Set Maximum Prime Value:
- The variable $max is set to 105000, which will be the upper limit for the prime numbers to be calculated.
- Create Prime Flag Array:
- A fixed-size array $arr is created using \SplFixedArray, with a size of max + 1. This array will hold flags indicating whether each number is prime.
- Initialize Array:
- A for loop initializes the array values from 2 to max to 1, indicating that all numbers are initially considered prime.
- Sieve of Eratosthenes Algorithm:
- The algorithm starts with i set to 2 and loops up to the square root of $max.
- If the current number is marked as prime (i.e., arr[$i] is 1), it enters another loop to mark its multiples as non-prime (0).
- This is done by iterating j and setting arr[$i * $j] to 0 for multiples of i.
- Input Processing Loop:
- The code reads input from standard input until the input '0' is encountered.
- Each input line is trimmed and converted to an integer $n.
- Initialize Variables for Summation:
- Two variables, $result and $cnt, are initialized to 0. $result will store the sum of prime numbers, and $cnt will count how many prime numbers have been found.
- • Find and Sum First N Prime Numbers:
- A for loop iterates through the array of numbers from 2 to max.
- If the count of found primes ($cnt) equals $n, the loop breaks.
- If the current number is prime (i.e., arr[$i] is 1), it is added to $result, and $cnt is incremented.
- Output the Result:
- After summing the required prime numbers, the code outputs the result, indicating the sum of the first n prime numbers.
Sample Input:
25
0
Sample Output:
Sum of first 25 prime numbers:1060
Flowchart:
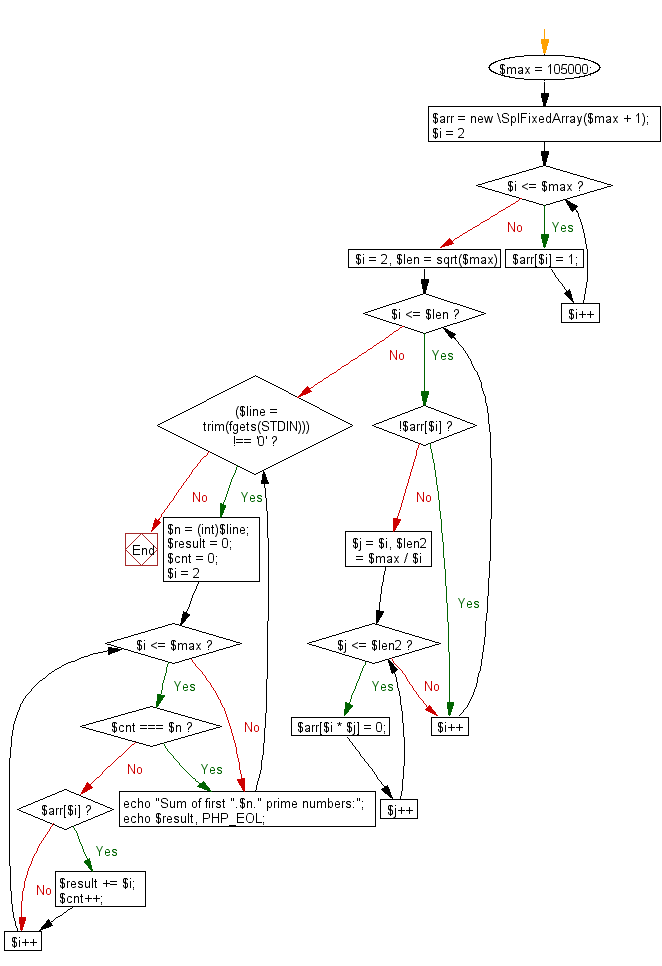
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a PHP program to replace a string "Python" with "PHP" and "Python" with " PHP" in a given string.
Next: Write a PHP program that accept a even number (n should be greater than or equal to 4 and less than or equal to 50000, Goldbach number) from the user and create a combinations that express the given number as a sum of two prime numbers. Print the number of combinations.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics