PHP Exercises: Replace a string "Python" with "PHP" and "Python" with "PHP" in a given string
63. Swap PHP and Python
Write a PHP program to replace a string "Python" with "PHP" and "Python" with " PHP" in a given string.
Input: English letters (including single byte alphanumeric characters, blanks, symbols) are given on one line. The length of the input character string is 1000 or less.
Pictorial Presentation:
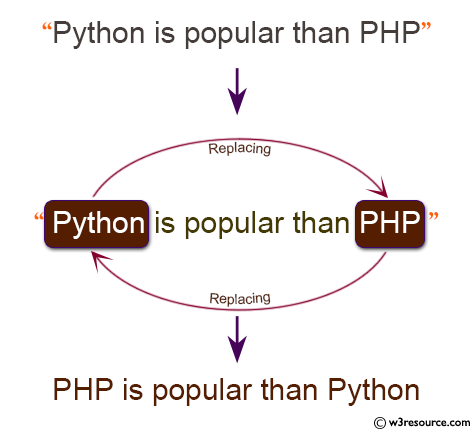
Sample Solution:
PHP Code:
<?php
// Read input from standard input and remove leading/trailing whitespaces
$str = trim(fgets(STDIN));
// Replace occurrences of 'Python' with '@ython' and 'PHP' with 'Python'
$str = str_replace(array('Python', 'PHP'), array('@ython', 'Python'), $str);
// Replace '@ython' with 'PHP' to get the final result
echo str_replace('@ython', 'PHP', $str), PHP_EOL;
?>
Explanation:
- Read Input:
- The code reads a line of input from standard input and removes any leading or trailing whitespace using trim(fgets(STDIN)).
- Replace Words:
- It uses str_replace to perform multiple replacements:
- It replaces occurrences of the string 'Python' with '@ython'.
- It replaces occurrences of the string 'PHP' with 'Python'.
- Final Replacement:
- After the initial replacements, it performs a second str_replace to replace '@ython' with 'PHP'.
- Output the Result:
- Finally, it outputs the modified string with echo, followed by a newline (PHP_EOL).
Sample Input:
PHP is popular than Python
Sample Output:
Python is popular than PHP.
Flowchart:
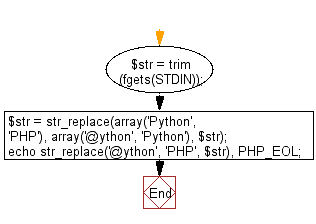
For more Practice: Solve these Related Problems:
- Write a PHP script to swap the occurrences of "PHP" and "Python" in a string using temporary placeholders.
- Write a PHP script to exchange two keywords in a given sentence without performing sequential replacements.
- Write a PHP function to replace "PHP" with "Python" and vice versa ensuring overlapping replacements are handled.
- Write a PHP script to perform a bidirectional swap on two substrings within a string dynamically.
Go to:
PREV : Parallelogram Type Check.
NEXT : Difference Between Largest and Smallest Integer Formed.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.