PHP Exercises: Reads a date and prints the day of the date
59. Find Day of the Date in 2016
Write a PHP program to that reads a date (from 2004/1/1 to 2004/12/31) and prints the day of the date. Jan. 1, 2004, is Friday. Note that 2004 is a leap year.
Two integers m and d separated by a single space in a line, m ,d represent the month and the day.
Input:Input numbers (real numbers) are separated by a space.
Input 0 to exit.
Visual Presentation:
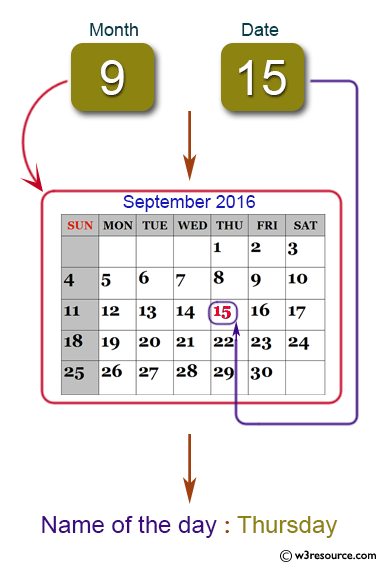
Sample Solution:
PHP Code:
<?php
// Define an array of days in a non-leap year
$days = explode(',', '0,31,60,91,121,152,182,213,244,274,305,335');
// Define an array of corresponding day names
$format = explode(',', 'Wednesday,Thursday,Friday,Saturday,Sunday,Monday,Tuesday');
// Continue reading lines from standard input until an empty line is encountered
while (($line = trim(fgets(STDIN))) !== '') {
// Extract month and day values from the input line
sscanf($line, '%d %d', $m, $d);
// Check if the month is 0, which indicates the end of input
if ($m === 0) {
break;
}
// Calculate the day of the year using the pre-defined array
$n = $days[$m - 1] + $d;
// Determine the day of the week using the pre-defined array of day names
$today = $format[$n % 7];
// Output the result
echo "The day is: " . $today, PHP_EOL;
}
?>
Explanation:
- Define Days Array:
- An array named $days is defined using explode(), representing the cumulative number of days at the end of each month in a non-leap year.
- The values are:
- January: 0
- February: 31
- March: 60
- April: 91
- May: 121
- June: 152
- July: 182
- August: 213
- September: 244
- October: 274
- November: 305
- December: 335
- Define Day Names Array:
- An array named $format is defined, which contains the names of the days of the week, starting from Wednesday.
- Read Input Until Empty Line:
- A while loop continues to read lines from standard input until an empty line is encountered.
- Extract Month and Day:
- The input line is parsed using sscanf() to extract the month ($m) and day ($d) values.
- Check for End of Input:
- If the month is 0, the loop breaks, indicating the end of input.
- Calculate Day of the Year:
- The day of the year ($n) is calculated by adding the cumulative days of the month (from the $days array) to the day of the month ($d).
- Determine Day of the Week:
- The day of the week is determined by taking the modulus of $n with 7 and using it to index into the $format array.
- Output the Result:
- The program outputs the day of the week corresponding to the given month and day.
Sample Input:
9 15
Sample Output:
The day is: Thursday
Flowchart:
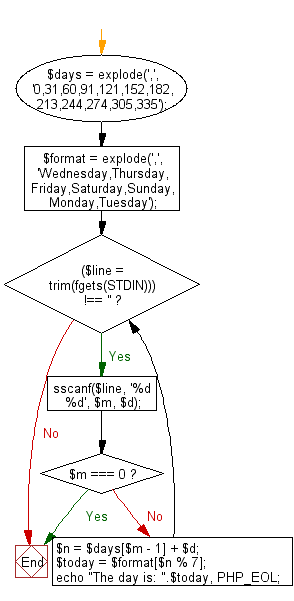
For more Practice: Solve these Related Problems:
- Write a PHP script to compute the weekday for any given date in 2016 using Zeller’s congruence.
- Write a PHP function to determine the day of the week for a date in a leap year and output the day name.
- Write a PHP script to compare computed weekdays with a preset 2016 calendar for verification.
- Write a PHP script to validate a 2016 date input and output the corresponding day of the week accurately.
Go to:
PREV : Circle Relationship.
NEXT : Mode Values from Sequence.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.