PHP Exercises: Test if circumference of two circles intersect or overlap
58. Circle Relationship
There are two circles C1 with radius r1, central coordinate (x1, y1) and C2 with radius r2 and central coordinate (x2, y2)
Write a PHP program to test the followings -
"C2 is in C1" if C2 is in C1
"C1 is in C2" if C1 is in C2
"Circumference of C1 and C2 intersect" if circumference of C1 and C2 intersect, and and
"C1 and C2 do not overlap" if C1 and C2 do not overlap.
Input:Input numbers (real numbers) are separated by a space.
Input 0 to exit.
Sample Solution:
PHP Code:
<?php
// Read the number of test cases from standard input
$n = intval(fgets(STDIN));
// Loop through each test case
for ($i = 0; $i < $n; $i++) {
// Read the coordinates and radii of two circles from standard input
fscanf(STDIN, "%lf %lf %lf %lf %lf %lf", $xa, $ya, $ra, $xb, $yb, $rb);
// Calculate the distance between the centers of the two circles
$r = sqrt(($xb - $xa) * ($xb - $xa) + ($yb - $ya) * ($yb - $ya));
// Check if one circle is completely inside the other
if ($r + $ra < $rb) {
echo "C1 is in C2\n";
continue;
}
if ($r + $rb < $ra) {
echo "C2 is in C1.\n";
continue;
}
// Check if the circumferences of the circles intersect
if ($r <= $ra + $rb) {
echo "Circumference of C1 and C2 intersect.\n";
continue;
}
// Circles do not overlap or intersect
echo "C1 and C2 do not overlap.\n";
}
?>
Explanation:
- Read Number of Test Cases:
- The first line reads an integer from standard input, which represents the number of test cases ($n).
- Loop Through Each Test Case:
- A for loop iterates from 0 to n-1, processing each test case.
- Read Circle Data:
- For each test case, the coordinates and radii of two circles are read from standard input using fscanf().
- Variables $xa, $ya, $ra represent the center coordinates and radius of Circle 1 (C1).
- Variables $xb, $yb, $rb represent the center coordinates and radius of Circle 2 (C2).
- Calculate Distance Between Centers:
- The distance between the centers of the two circles is calculated using the distance formula: r=(xb−xa)2+(yb−ya)2r = \sqrt{(xb - xa)^2 + (yb - ya)^2}r=(xb−xa)2+(yb−ya)2
- Check If One Circle Is Inside the Other:
- Circle 1 inside Circle 2: If the sum of the radius of Circle 1 and the distance between the centers is less than the radius of Circle 2, it outputs "C1 is in C2".
- Circle 2 inside Circle 1: If the sum of the radius of Circle 2 and the distance is less than the radius of Circle 1, it outputs "C2 is in C1".
- Check If Circles Intersect:
- If the distance between the centers is less than or equal to the sum of the radii of both circles, it outputs "Circumference of C1 and C2 intersect".
- Circles Do Not Overlap:
- If none of the previous conditions are met, it outputs "C1 and C2 do not overlap".
Sample Input:
2
0.0 0.0 6.0 0.0 0.0 5.0
0.0 0.0 3.0 5.1 0.0 3.0
Sample Output:
C2 is in C1. Circumference of C1 and C2 intersect.
Flowchart:
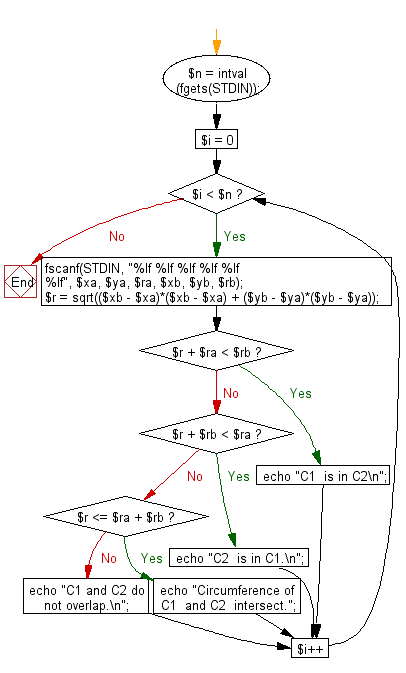
For more Practice: Solve these Related Problems:
- Write a PHP script to determine the spatial relationship between two circles based on their centers and radii.
- Write a PHP function to compute the distance between circle centers and compare it with the radii to decide overlap type.
- Write a PHP script to analyze whether one circle is fully inside another or if their circumferences intersect.
- Write a PHP script to output specific relationship messages by comparing circle distances and radius differences.
Go to:
PREV : Maximum Sum of Contiguous Subsequence.
NEXT : Find Day of the Date in 2016.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.