PHP Exercises: Test whether two lines PQ and RS are parallel
56. Test Parallel Lines
Write a PHP program to test whether two lines PQ and RS are parallel.
The four points are P(x1, y1), Q(x2, y2), R(x3, y3), S(x4, y4).
Input: −100 ≤ x1, y1, x2, y2, x3, y3, x4, y4 ≤ 100
Each value is a real number with at most 5 digits after the decimal point.
Sample Solution:
PHP Code:
<?php
// Read the number of test cases from standard input
fscanf(STDIN, '%d', $n);
// Loop through each test case
for ($i = 0; $i < $n; $i++) {
// Read the coordinates of two points (x1, y1) and (x2, y2) for segment PQ
fscanf(STDIN, '%f %f %f %f', $x1, $y1, $x2, $y2);
// Read the coordinates of two points (x3, y3) and (x4, y4) for segment RS
fscanf(STDIN, '%f %f %f %f', $x3, $y3, $x4, $y4);
// Calculate the slopes of segments PQ and RS
$pq = INF;
if ($x2 - $x1 !== 0.0) {
$pq = ($y2 - $y1) / ($x2 - $x1);
}
$rs = INF;
if ($x4 - $x3 !== 0.0) {
$rs = ($y4 - $y3) / ($x4 - $x3);
}
// Check if the slopes are equal to determine if PQ and RS are parallel
echo $pq === $rs ? 'PQ and RS are parallel.' : 'PQ and RS are not parallel.';
echo PHP_EOL;
}
?>
Explanation:
- Read Number of Test Cases:
- The code reads an integer value from standard input, representing the number of test cases, using fscanf().
- Loop Through Test Cases:
- A for loop iterates through each test case based on the number of test cases read.
- Read Coordinates for Segment PQ:
- Inside the loop, the coordinates of two points, (x1,y1)(x1, y1)(x1,y1) and (x2,y2)(x2, y2)(x2,y2), are read from standard input for segment PQ using fscanf().
- Read Coordinates for Segment RS:
- The coordinates of two points, (x3,y3)(x3, y3)(x3,y3) and (x4,y4)(x4, y4)(x4,y4), are similarly read for segment RS.
- Calculate Slopes of Segments:
- The slope of segment PQ is calculated. If x2−x1x2 - x1x2−x1 is not zero, the slope is calculated using the formula slope=y2−y1x2−x1\text{slope} = \frac{y2 - y1}{x2 - x1}slope=x2−x1y2−y1; otherwise, it is set to INF (infinity).
- The slope of segment RS is calculated in the same way.
- Check if Segments are Parallel:
- The code checks if the slopes of PQ and RS are equal. If they are equal, it indicates that the segments are parallel; otherwise, they are not.
- Output Result:
- The result of the comparison is printed to standard output, stating whether "PQ and RS are parallel" or "PQ and RS are not parallel", followed by a newline using PHP_EOL.
Input:
2
1.0 0.0 3.0 2.0 2.0 2.0 0.0 0.0
4.0 3.0 10.0 7.0 14.0 5.0 8.0 10.0
Sample Output:
PQ and RS are parallel. PQ and RS are not parallel.
Flowchart:
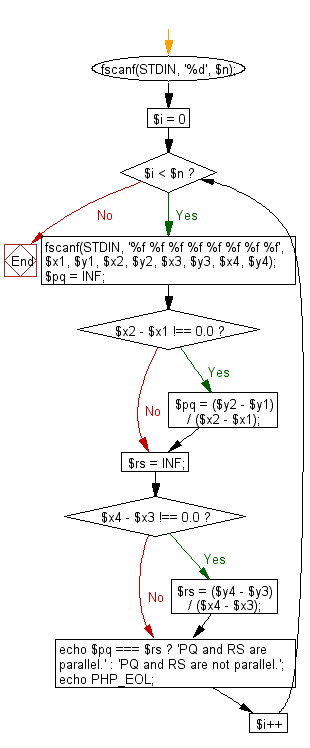
For more Practice: Solve these Related Problems:
- Write a PHP script to determine if two lines are parallel by comparing their slopes.
- Write a PHP function to compute line slopes from coordinate pairs and verify parallelism.
- Write a PHP script to check parallel lines by calculating the slopes and ensuring equality.
- Write a PHP script to validate line equations from input coordinates and determine if they are parallel.
Go to:
PREV : Sort Six Numbers Descending.
NEXT : Maximum Sum of Contiguous Subsequence.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.