PHP Exercises: Compute and print sum of two given integers
PHP: Exercise-54 with Solution
Write a PHP program to compute and print sum of two given integers (more than or equal to zero). If given integers or the sum have more than 80 digits, print "overflow".
Pictorial Presentation:
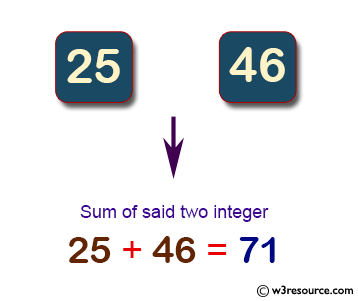
Sample Solution:
PHP Code:
<?php
// Read the number of test cases
fscanf(STDIN, '%d', $N);
// Loop through each test case
for ($i = 0; $i < $N; $i++) {
// Read two numbers as strings
fscanf(STDIN, '%s', $a);
fscanf(STDIN, '%s', $b);
// Check if the length of either number is greater than 80
if (max(strlen($a), strlen($b)) > 80) {
echo "overflow\n";
continue; // Skip to the next iteration if overflow occurs
}
// Initialize an array to store the result
$arr = array_fill(0, 81, 0);
// Pad the numbers with leading zeros to make them of length 81
$a = sprintf('%081s', $a);
$b = sprintf('%081s', $b);
// Perform addition of the two numbers
for ($j = 80; $j > 0; $j--) {
$n = $arr[$j] + $a[$j] + $b[$j];
// Check for carry
if ($n >= 10) {
$arr[$j] = substr($n, 1);
$arr[$j - 1] += 1;
} else {
$arr[$j] = $n;
}
}
// Remove leading zeros and check for overflow
$result = preg_replace('/^0+(\d+)$/', '$1', implode('', $arr));
// Output the result or indicate overflow
if (strlen($result) > 80) {
echo "overflow\n";
} else {
echo $result, PHP_EOL;
}
}
?>
Sample Output:
46 overflow overflow overflow overflow ... overflow overflow overflow
Flowchart:
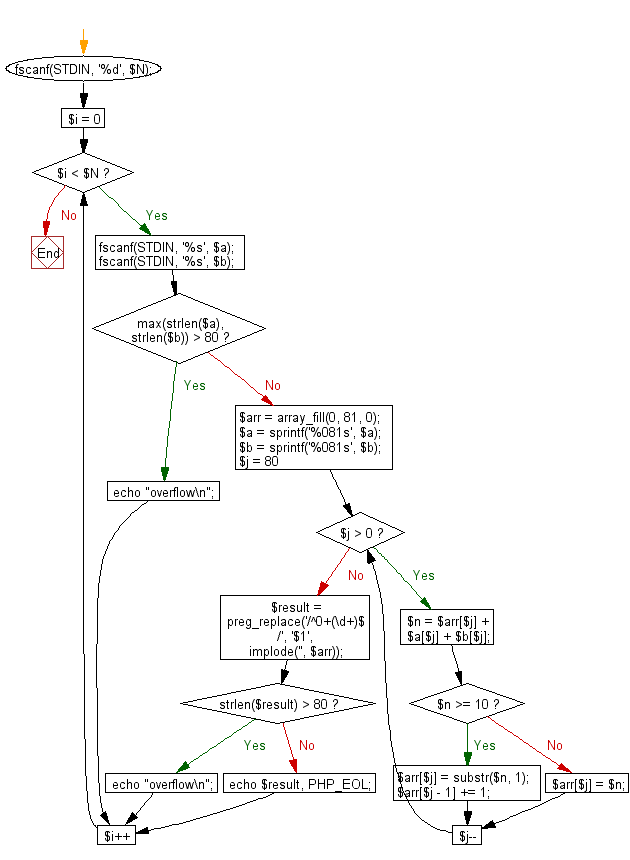
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a PHP program to compute the radius and the central coordinate (x, y) of a circle which is constructed by three given points on the plane surface.
Next: Write a PHP program that accepts six numbers as input and sorts them in descending order.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics