PHP Exercises: Print the number of prime numbers which are less than or equal to a given integer
PHP: Exercise-52 with Solution
Write a PHP program to print the number of prime numbers which are less than or equal to a given integer.
Input:
n (1 ≤ n ≤ 999,999) .
Pictorial Presentation:
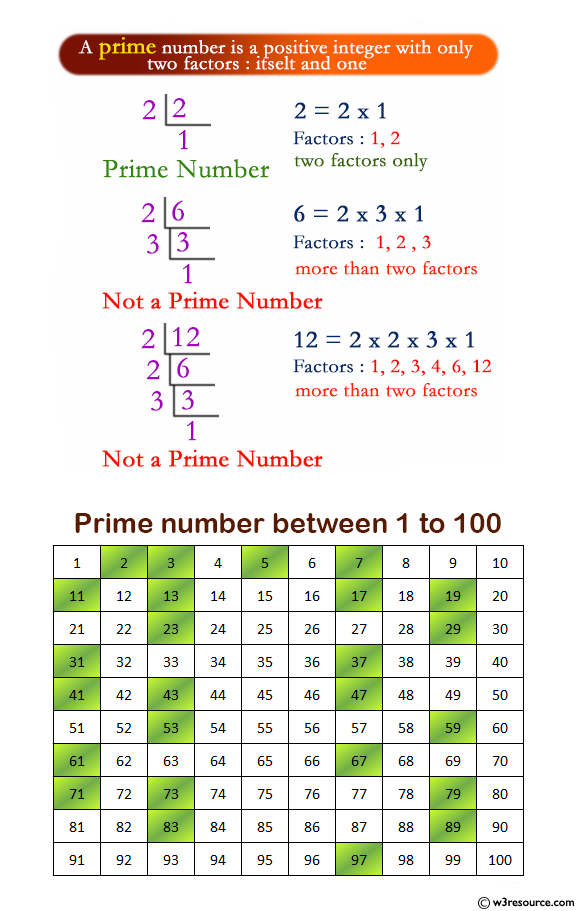
Sample Solution:
PHP Code:
<?php
// Initialize an array to mark numbers as prime or not (Sieve of Eratosthenes)
$a = array_fill(0, 1000000, true);
$a[0] = $a[1] = false;
// Iterate through numbers and mark multiples as non-prime
for ($i = 2; $i * $i < 1000000; $i++) {
if (!$a[$i]) continue;
for ($j = $i * $i; $j < 1000000; $j += $i) {
$a[$j] = false;
}
}
// Initialize an array to store the cumulative count of prime numbers
$sum = array_fill(0, 1000000, 0);
// Calculate the cumulative count of prime numbers up to each index
for ($i = 1; $i < 1000000; $i++) {
$sum[$i] += $sum[$i - 1];
if ($a[$i]) $sum[$i]++;
}
// Read input from standard input until the end of file
while (fscanf(STDIN, "%d", $n)) {
// Print the number of prime numbers which are less than or equal to n
echo "Number of prime numbers which are less than or equal to n: ";
echo $sum[$n] . "\n";
}
?>
Sample Output:
Sample Input: 50 Number of prime numbers which are less than or equal to n: 15
Flowchart:
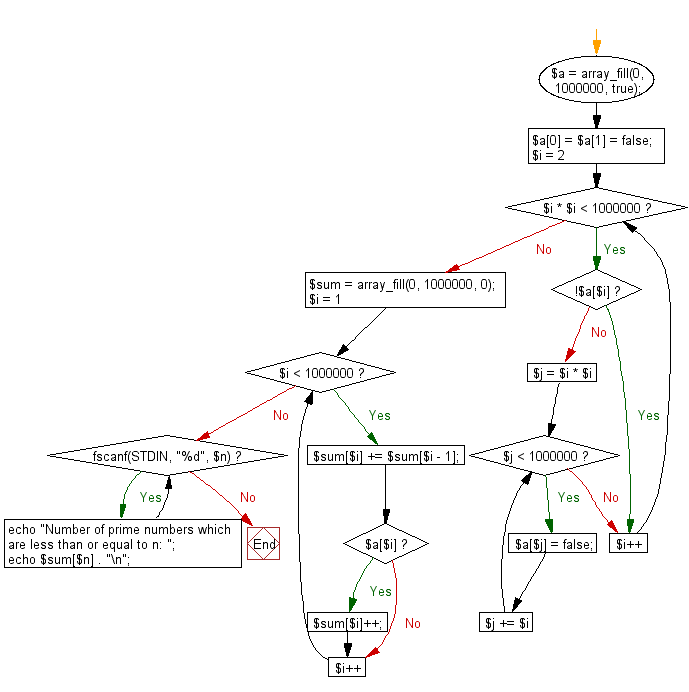
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a PHP program to compute the amount of the debt in n months.
Next: Write a PHP program to compute the radius and the central coordinate (x, y) of a circle which is constructed by three given points on the plane surface.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics