PHP Exercises: Compute the amount of the debt in n months
Write a PHP program to compute the amount of the debt in n months. The borrowing amount is $100,000 and the loan adds 5% interest of the debt and rounds it to the nearest 1,000 above month by month.
Input:
An integer n (0 ≤ n ≤ 100) .
Sample Solution:
PHP Code:
<?php
// Read an integer from standard input representing the number of years
fscanf(STDIN, '%d', $n);
// Initialize the initial debt amount
$debt = 100000;
// Loop through each year to calculate the debt amount after each year
for ($i = 0; $i < $n; $i++) {
// Calculate the new debt amount after applying a 5% increase and rounding to the nearest thousand
$debt = ceil(($debt * 1.05) / 1000) * 1000;
}
// Print the final amount of debt
echo "\nAmount of debt: ";
echo $debt . PHP_EOL;
?>
Explanation:
- Reading Input:
- The program reads an integer from standard input (STDIN) that represents the number of years ($n) using fscanf(STDIN, '%d', $n);.
- Initializing Debt:
- A variable $debt is initialized to 100,000, representing the starting amount of debt.
- Loop Through Years:
- A for loop runs from 0 to $n - 1, iterating over each year to calculate the debt for each year.
- Calculating New Debt:
- Inside the loop, the new debt amount is calculated by applying a 5% increase:
- ($debt * 1.05) calculates the increased debt.
- This amount is then divided by 1000, rounded up to the nearest whole number using ceil(), and multiplied back by 1000 to round to the nearest thousand.
- Outputting Final Debt:
- After the loop completes, the final amount of debt is printed to the standard output, preceded by the message "Amount of debt: ".
Output:
Amount of debt: 137000
Flowchart:
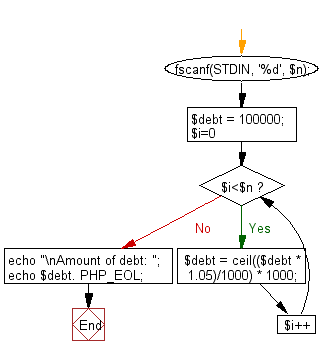
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a PHP program which solve the equation. Print the values of x, y where a, b, c, d, e and f are given.
Next: Write a PHP program which reads an integer n and find the number of combinations.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics