PHP Exercises: Compute the digit number of sum of two given integers
Write a PHP program to compute the digit number of sum of two given integers.
Input:
Each test case consists of two non-negative integers x and y which are separated by a space in a line.
0 ≤ x, y ≤ 1,000,000
Pictorial Presentation:
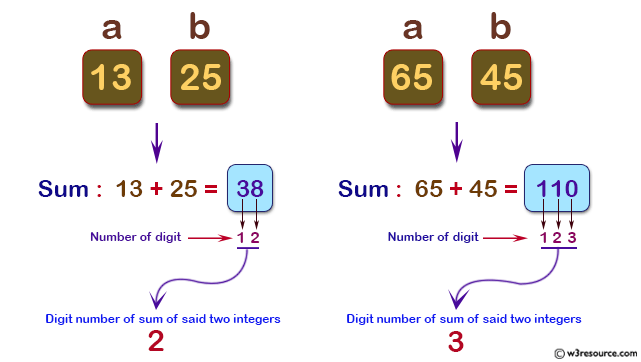
Sample Solution:
PHP Code:
<?php
// Infinite loop to continuously read input from STDIN
while (true) {
// Read and split the input line into an array of strings
$inputs = explode(' ', trim(fgets(STDIN)));
// Check if the input is not an array or has fewer than 2 elements
if (!is_array($inputs) || count($inputs) < 2) {
exit; // Exit the program if conditions are met
}
// Extract the first and second elements from the input array
$a = $inputs[0];
$b = $inputs[1];
// Call the numDigits function to calculate the number of digits in the sum
$d = numDigits($a + $b);
// Print the result with a descriptive message
echo("Digit number of sum of two given integers: ");
echo $d . "\n";
}
// Function to calculate the number of digits in a given number
function numDigits($n) {
return (int)(log10($n) + 1);
}
?>
Explanation:
- Infinite Loop:
- The code contains a while (true) loop that runs indefinitely to continuously read input from the user.
- Reading Input:
- Inside the loop, it reads a line from standard input (STDIN) using fgets(STDIN).
- The line is trimmed of whitespace and split into an array of strings using explode(' ', trim(...)).
- Input Validation:
- The code checks if the input is not an array or if it has fewer than two elements (count($inputs) < 2).
- If this condition is met, the program exits using exit.
- Extracting Values:
- The first and second elements of the input array are assigned to variables $a and $b, respectively.
- Calculating the Number of Digits:
- The code calls the numDigits function, passing the sum of $a and $b as an argument, to calculate the number of digits in the result.
- Printing the Result:
- It prints a message indicating that it will show the number of digits in the sum.
- The result ($d, the number of digits) is printed.
- Function Definition:
- The numDigits function calculates the number of digits in a given number $n.
- It uses the formula (int)(log10($n) + 1), which calculates the logarithm base 10 of $n to determine the number of digits.
Output:
Digit number of sum of two given integers: 2
Flowchart:
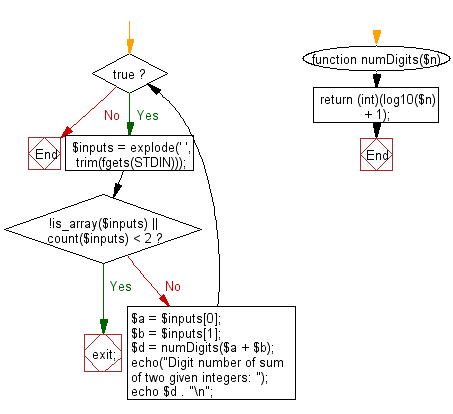
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a PHP program to find heights of the top three building in descending order from eight given buildings.
Next: Write a PHP program to check whether three given lengths (integers) of three sides form a right triangle. Print "Yes" if the given sides form a right triangle otherwise print "No".
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.