PHP Exercises: Find heights of the top three building in descending order from eight given buildings
46. Top Three Building Heights
Write a PHP program to find heights of the top three building in descending order from eight given buildings.
Input:
0 ≤ height of building (integer) ≤ 10,000
Pictorial Presentation:
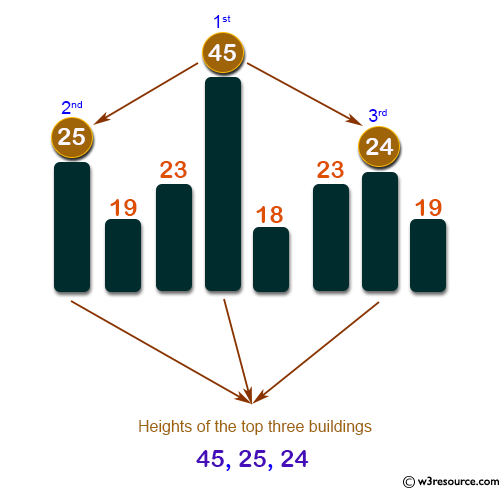
Sample Solution:
PHP Code:
<?php
// Create an empty array to store building heights
$heights = array();
// Read input from STDIN until end-of-file
while ($line = fgets(STDIN)) {
// Remove newline characters and convert to integer, then push to the heights array
rtrim($line, "\n");
array_push($heights, (int)$line);
}
// Sort the heights array in descending order
rsort($heights);
// Print the top three building heights
print("Heights of the top three buildings:\n");
echo $heights[0]."\n"; // Print the height of the tallest building
echo $heights[1]."\n"; // Print the height of the second tallest building
echo $heights[2]."\n"; // Print the height of the third tallest building
?>
Explanation:
- Array Initialization:
- An empty array called $heights is created to store the heights of buildings.
- Reading Input:
- A while loop is used to read input from the standard input (STDIN) line by line until the end-of-file (EOF) is reached.
- fgets(STDIN) reads a line of input.
- Processing Input:
- Each line is processed to remove any trailing newline characters using rtrim($line, "\n").
- The line is then converted to an integer using (int)$line and pushed into the $heights array using array_push().
- Sorting Heights:
- The rsort($heights) function sorts the $heights array in descending order, meaning the tallest buildings will appear first.
- Output Top Three Heights:
- A message is printed to indicate that the top three building heights will be displayed.
- The first three elements of the sorted $heights array (i.e., the tallest, second tallest, and third tallest buildings) are printed using echo.
- Prints:
- echo $heights[0]."\n" prints the height of the tallest building.
- echo $heights[1]."\n" prints the height of the second tallest building.
- echo $heights[2]."\n" prints the height of the third tallest building.
Output:
Heights of the top three buildings: 45 25 24
Flowchart:
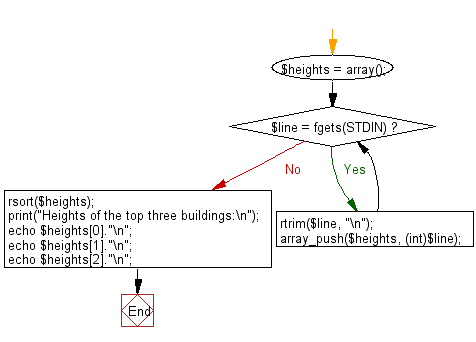
For more Practice: Solve these Related Problems:
- Write a PHP script to extract the top three maximum values from an array of building heights.
- Write a PHP script to sort an array of building heights and output the three tallest values.
- Write a PHP function to retrieve three unique maximum heights from a list using custom sorting.
- Write a PHP script to filter building heights and display the top three using an optimized algorithm.
Go to:
PREV : Sum of Digits.
NEXT : Digit Count of Sum.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.