PHP Exercises: Find the first non-repeated character in a given string
42. First Non-Repeated Character
Write a PHP program to find the first non-repeated character in a given string.
Sample Example:
Input: Green
Output: G
Input: abcdea
Output: b
Sample Solution:
PHP Code:
<?php
// Define a function to find the first non-repeating character in a word
function find_non_repeat($word) {
$chr = null;
// Iterate through each character in the word
for ($i = 0; $i <= strlen($word); $i++) {
// Check if the count of the current character is 1 (non-repeating)
if (substr_count($word, substr($word, $i, 1)) == 1) {
// Return the first non-repeating character found
return substr($word, $i, 1);
}
}
}
// Test the function with different words
echo find_non_repeat("Green")."\n"; // Output: G
echo find_non_repeat("abcdea")."\n"; // Output: b
?>
Explanation:
- Define Function find_non_repeat($word):
- This function finds and returns the first non-repeating character in a given string $word.
- Initialize Variable:
- $chr is defined but not used (possibly intended to store the result, though it's unnecessary here).
- Loop Through Each Character:
- for ($i = 0; $i <= strlen($word); $i++) iterates over each character in $word.
- Check for Non-Repeating Character:
- substr_count($word, substr($word, $i, 1)) == 1 checks if the current character appears only once in the string.
- Return First Non-Repeating Character:
- If a non-repeating character is found, return substr($word, $i, 1) immediately returns it, ending the function.
- Test Cases:
- find_non_repeat("Green") outputs "G", as it's the first unique character.
- find_non_repeat("abcdea") outputs "b", the first non-repeating character in this word.
Output:
G b
Flowchart:
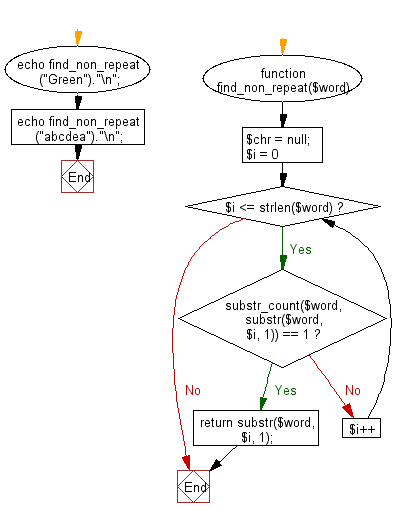
For more Practice: Solve these Related Problems:
- Write a PHP function to find the first non-repeated character in a string using associative arrays.
- Write a PHP script that scans a string with repeated characters and returns the first unique character.
- Write a PHP script to determine the first non-repeating character in a multi-byte Unicode string.
- Write a PHP script to optimize the search for the first unique character in very long strings.
Go to:
PREV : Multiplication Table 6x6.
Next: Multiply Corresponding List Elements.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.