PHP Exercises: Print out the multiplication table upto 6*6
41. Multiplication Table 6x6
Write a PHP program to print out the multiplication table upto 6*6.
Sample Solution:
PHP Code:
<?php
// Outer loop for rows
for ($i = 1; $i < 7; $i++) {
// Inner loop for columns
for ($j = 1; $j < 7; $j++) {
// Check if it's the first column
if ($j == 1) {
// Display the product of $i and $j, left-padded to 2 characters
echo str_pad($i * $j, 2, " ", STR_PAD_LEFT);
} else {
// Display the product of $i and $j, left-padded to 4 characters
echo str_pad($i * $j, 4, " ", STR_PAD_LEFT);
}
}
// Move to the next line after each row is printed
echo "\n";
}
?>
Explanation:
- Outer Loop for Rows:
- for ($i = 1; $i < 7; $i++) iterates from 1 to 6, representing each row.
- Inner Loop for Columns:
- for ($j = 1; $j < 7; $j++) iterates from 1 to 6 for each column within the current row.
- Display Values Based on Column Position:
- If $j == 1 (first column), it displays the product of $i * $j, left-padded to 2 characters.
- For other columns ($j > 1), it displays the product of $i * $j, left-padded to 4 characters.
- End of Row:
- echo "\n" moves to the next line after each row is printed, creating a new row in the output.
Output:
1 2 3 4 5 6 2 4 6 8 10 12 3 6 9 12 15 18 4 8 12 16 20 24 5 10 15 20 25 30 6 12 18 24 30 36
Flowchart:
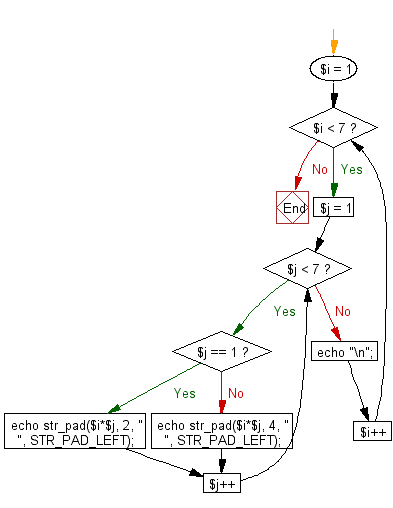
For more Practice: Solve these Related Problems:
- Write a PHP script to output a formatted 6x6 multiplication table using HTML table tags.
- Write a PHP script to generate a 6x6 multiplication grid using nested loops and store the results in a multidimensional array.
- Write a PHP script to create a multiplication table that highlights products which are prime numbers.
- Write a PHP script to print a multiplication table with custom spacing and border characters for clarity.
Go to:
PREV : Calculate Modulus Without %.
NEXT : First Non-Repeated Character.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.