PHP Exercises: Calculate the mod of two given integers without using any inbuilt modulus operator
Write a PHP program to calculate the mod of two given integers without using any inbuilt modulus operator.
Sample Solution:
PHP Code:
<?php
// Function to calculate the remainder without using the modulus operator
function without_mod($m, $n)
{
// Calculate how many times $n divides into $m
$divides = (int) ($m / $n);
// Calculate the remainder without using the modulus operator
return $m - $n * $divides;
}
// Example usage of the function with inputs (13, 2)
echo without_mod(13, 2) . "\n";
// Example usage of the function with inputs (81, 3)
echo without_mod(81, 3) . "\n";
?>
Explanation:
- Define a Function to Calculate Remainder Without Using the Modulus Operator:
- function without_mod($m, $n) defines a function that takes two integers, $m and $n, and calculates the remainder of $m divided by $n without using %.
- Calculate Division Result:
- (int) ($m / $n) calculates how many times $n divides into $m and stores it in $divides.
- Calculate Remainder:
- $m - $n * $divides returns the remainder by subtracting the product of $n and $divides from $m.
- Test the Function:
- echo without_mod(13, 2) prints the remainder of 13 / 2, which is 1.
- echo without_mod(81, 3) prints the remainder of 81 / 3, which is 0.
Output:
1 0
Flowchart:
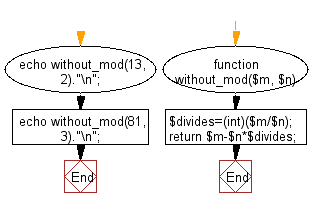
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a PHP program to get the size of a file.
Next: Write a PHP program to print out the multiplication table upto 6*6.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics