PHP Exercises: Valid an email address
Write a PHP program to valid an email address.
Sample Solution:
PHP Code:
<?php
// Function to validate an email address
function valid_email($email)
{
// Trim any leading or trailing whitespaces from the email
$result = trim($email);
// Check if the trimmed email is a valid email address
if (filter_var($result, FILTER_VALIDATE_EMAIL))
{
// If valid, return "true"
return "true";
}
else
{
// If not valid, echo "false"
echo "false";
}
}
// Test the function with valid and invalid email addresses
echo valid_email("[email protected]") . "\n";
echo valid_email("abc#example.com") . "\n";
?>
Explanation:
- Define Function valid_email:
- The function valid_email($email) checks if the provided email address is valid.
- Trim Whitespace:
- trim($email) removes any leading or trailing whitespace from the email input and assigns it to $result.
- Validate Email Format:
- filter_var($result, FILTER_VALIDATE_EMAIL) checks if $result is a valid email address format.
- If valid, the function returns "true".
- If invalid, it prints "false".
- Test Cases:
- The function is tested with "[email protected]" (valid) and "abc#example.com" (invalid). The results are printed.
Output:
true false
Flowchart:
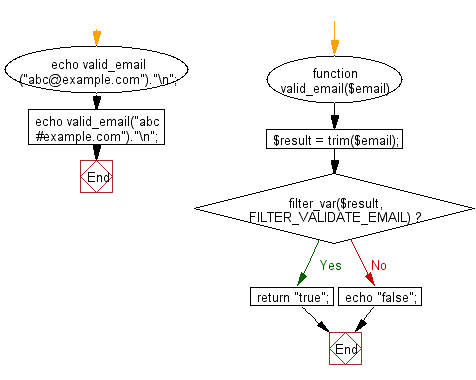
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a PHP program to compute the sum of the prime numbers less than 100.
Next: Write a PHP program to get the size of a file.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics