PHP Exercises: Test if a given string occurs at the end of another given string
Write a PHP program to test if a given string occurs at the end of another given string.
Sample Solution:
PHP Code:
<?php
// Function to check if str2 is at the end of str1
function str2_in_str1($str1, $str2) {
// Calculate the length of str2
$p_len = strlen($str2);
// Calculate the length of str1
$w_len = strlen($str1);
// Calculate the starting index for str2 in str1
$w_start = $w_len - $p_len - 1;
// Check if the substring at the calculated index in str1 is equal to str2
if (substr($str1, $w_len - $p_len, $p_len) == $str2) {
return "true";
} else {
return "false";
}
}
// Example usage with different strings
echo str2_in_str1("Python", "on") . "\n"; // Output: true
echo str2_in_str1("JavaScript", "php") . "\n"; // Output: false
?>
Explanation:
- Function Definition:
- The function str2_in_str1($str1, $str2) checks if the string $str2 appears at the end of the string $str1.
- Calculate Lengths:
- $p_len stores the length of $str2.
- $w_len stores the length of $str1.
- Determine Starting Index:
- $w_start calculates the index where $str2 should start in $str1 if it appears at the end. This is calculated by subtracting $p_len from $w_len.
- Check if End of $str1 Matches $str2:
- substr($str1, $w_len - $p_len, $p_len) extracts the substring from $str1 that has the same length as $str2 and starts at the calculated position.
- If this substring matches $str2, the function returns "true", indicating $str2 is at the end of $str1; otherwise, it returns "false".
- Example Usage:
- The function is tested with "Python", "on" (returns "true") and "JavaScript", "php" (returns "false"), and the results are printed.
Output:
true false
Flowchart:
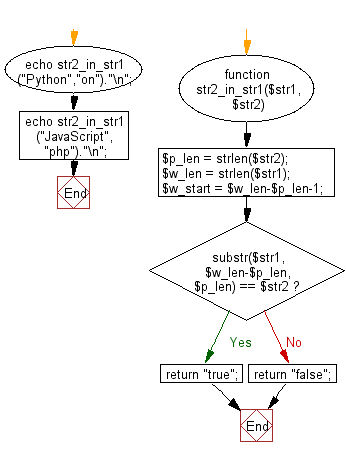
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a PHP program to remove duplicates from a sorted list.
Next: Write a PHP program to compute the sum of the prime numbers less than 100.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics