PHP Exercises: Remove duplicates from a sorted list
PHP: Exercise-35 with Solution
Write a PHP program to remove duplicates from a sorted list.
Input: (1,1,2,2,3,4,5,5)
Output: (1,2,3,4,5)
Sample Solution:
PHP Code:
<?php
// Function to remove duplicates from a list and return unique values
function remove_duplicates_list($list1) {
// Use array_unique to get unique values and array_values to re-index the array
$nums_unique = array_values(array_unique($list1));
return $nums_unique;
}
// Example usage with an array containing duplicate values
$nums = array(1, 1, 2, 2, 3, 4, 5, 5);
// Call the function and print the result
print_r(remove_duplicates_list($nums));
?>
Sample Output:
Array ( [0] => 1 [1] => 2 [2] => 3 [3] => 4 [4] => 5 )
Flowchart:
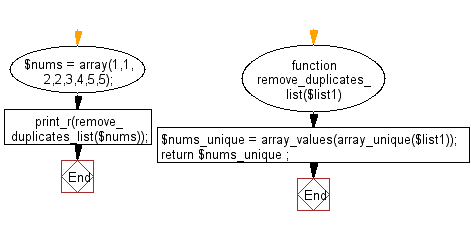
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a PHP program to check if the bits of the two given positions of a number are same or not.
Next: Write a PHP program to test if a given string occurs at the end of another given string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics