PHP Exercises: Check if the bits of the two given positions of a number are same or not
PHP: Exercise-34 with Solution
Write a PHP program to check if the bits of the two given positions of a number are same or not.
112 - > 01110000
Test 2nd and 3rd position
Result: True
Test 4th and 5th position
Result: False
Sample Solution:
PHP Code:
<?php
// Function to test if bits at two specified positions are equal
function test_bit_position($num, $pos1, $pos2) {
// Adjust positions to start from 0 (as arrays in PHP are 0-indexed)
$pos1--;
$pos2--;
// Convert the decimal number to a binary string and reverse it
$bin_num = strrev(decbin($num));
// Check if the bits at the specified positions are equal
if ($bin_num[$pos1] == $bin_num[$pos2]) {
return "true";
} else {
return "false";
}
}
// Test case
echo test_bit_position(112, 5, 6) . "\n";
?>
Sample Output:
true
Flowchart:
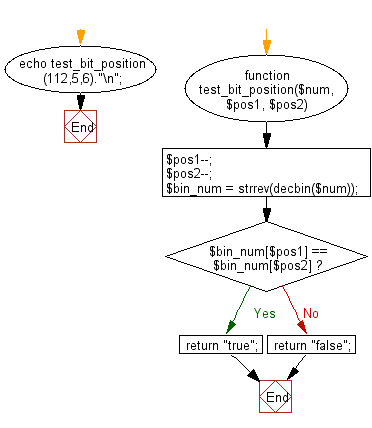
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a PHP program to convert word to digit.
Next: Write a PHP program to remove duplicates from a sorted list.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics