PHP Exercises : Swap two variables
Write a PHP program to swap two variables.
PHP: Swapping two variables
Swapping two variables refers to mutually exchanging the values of the variables. Generally, this is done with the data in memory.
The simplest method to swap two variables is to use a third temporary variable :
define swap(a, b) temp := a a := b b := temp
Sample Solution:
Code : Swap two numbers using a third variable
PHP Code:
<?php
// Declare and initialize two variables
$a = 15;
$b = 27;
// Display the original values of the variables
echo "\nThe number before swapping is:\n";
echo "Number a =" . $a . " and b=" . $b;
// Swap Logic
$temp = $a;
$a = $b;
$b = $temp;
// Display the values after swapping
echo "\nThe number after swapping is: \n";
echo "Number a =" . $a . " and b=" . $b . "\n";
?>
Code: Swap two variables value without using third variable
<?php
// Declare and initialize two variables
$a = 15;
$b = 276;
// Display the values before swapping
echo "\nBefore swapping: ". $a . ',' . $b;
// Swap Logic using list() and array()
list($a, $b) = array($b, $a);
// Display the values after swapping
echo "\nAfter swapping: ". $a . ',' . $b."\n";
?>
Explanation:
- Variable Declaration and Initialization:
- Two variables, $a and $b, are declared and initialized with the values 15 and 27, respectively.
- Display Original Values:
- The code uses echo to output the original values of $a and $b, prefixed by the message "The number before swapping is:".
- Swap Logic:
- A temporary variable $temp is used to facilitate the swapping of values:
- The value of $a is stored in $temp.
- The value of $b is assigned to $a.
- The value stored in $temp is then assigned to $b.
- After this operation, $a now holds the value 27, and $b holds the value 15.
- Display Values After Swapping:
- The code uses echo to output the new values of $a and $b, prefixed by the message "The number after swapping is:".
Output:
The number before swapping is: Number a =15 and b=27 The number after swapping is: Number a =27 and b=15
Flowchart:
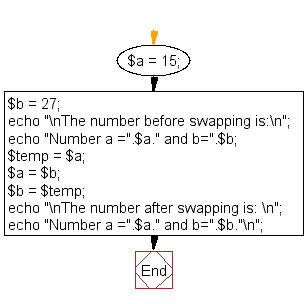
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a PHP script to get the time of the last modification of the current page.
Next: Write a PHP program to check if a number is an Armstrong number or not. Return true if the number is Armstrong otherwise return false.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.