PHP Exercises : Get the names of the functions of a module
PHP : Exercise-29 with Solution
Write a PHP script to get the names of the functions of a module.
Note : Find XML, JSON functions
Sample Solution:
PHP Code:
<?php
// Get the names of all functions provided by the JSON extension
$json_extension_funcs = get_extension_funcs("JSON");
// Print the list of JSON extension functions
print_r($json_extension_funcs);
echo "\n";
// Get the names of all functions provided by the XML extension
$xml_extension_funcs = get_extension_funcs("XML");
// Print the list of XML extension functions
print_r($xml_extension_funcs);
echo "\n";
?>
Sample Output:
Array ( [0] => json_encode [1] => json_decode [2] => json_last_error [3] => json_last_error_msg ) Array ( [0] => xml_parser_create [1] => xml_parser_create_ns [2] => xml_set_object [3] => xml_set_element_handler [4] => xml_set_character_data_handler [5] => xml_set_processing_instruction_handler [6] => xml_set_default_handler [7] => xml_set_unparsed_entity_decl_handler [8] => xml_set_notation_decl_handler [9] => xml_set_external_entity_ref_handler [10] => xml_set_start_namespace_decl_handler [11] => xml_set_end_namespace_decl_handler [12] => xml_parse [13] => xml_parse_into_struct [14] => xml_get_error_code [15] => xml_error_string [16] => xml_get_current_line_number [17] => xml_get_current_column_number [18] => xml_get_current_byte_index [19] => xml_parser_free [20] => xml_parser_set_option [21] => xml_parser_get_option [22] => utf8_encode [23] => utf8_decode )
Flowchart:
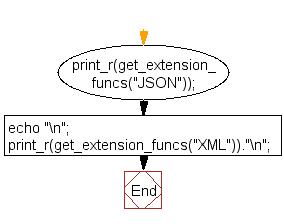
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a PHP script to get the directory path used for temporary files.
Next: Write a PHP script to get the time of the last modification of the current page.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics