PHP Exercises : Get the information about the OS PHP is running on
Write a PHP script to get the information about the operating system PHP is running on.
Sample Solution:
PHP Code:
<?php
// Get and echo the system name, version, and machine type using php_uname()
echo php_uname() . "\n";
// Echo the operating system PHP is running on
echo PHP_OS . "\n";
// Check if the operating system is Windows using the PHP_OS constant
if (strtoupper(substr(PHP_OS, 0, 3)) === 'WIN') {
echo 'This is a server using Windows!';
} else {
echo 'This is a server not using Windows!' . "\n";
}
?>
Explanation:
- Get System Information:
- The php_uname() function retrieves information about the operating system PHP is running on, including the system name, version, and machine type.
- The result is output using echo, followed by a newline (\n).
- Output Operating System:
- PHP_OS is a predefined constant in PHP that returns the name of the operating system (e.g., Linux, WINNT).
- This value is echoed to display the operating system PHP is running on, followed by a newline.
- Check for Windows Operating System:
- The code checks if the operating system is Windows by using strtoupper(substr(PHP_OS, 0, 3)) to get the first three letters of PHP_OS and convert them to uppercase.
- If the result equals 'WIN', it outputs 'This is a server using Windows!'.
- Otherwise, it outputs 'This is a server not using Windows!', followed by a newline.
Output:
Linux programming-editor 4.4.0-78-generic #99-Ubuntu SMP Thu Apr 27 15:29:09 UTC 2017 x86_64 Linux This is a server not using Windows!
Flowchart:
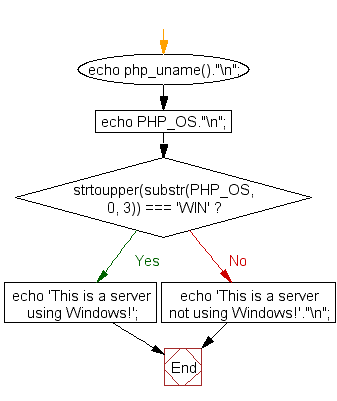
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a PHP script to get the document root directory under which the current script is executing, as defined in the server's configuration file.
Next: Write a PHP script to print out all the credits for PHP.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics