PHP Exercises : Compare the PHP version
23. Compare PHP Version
Write a PHP script to compare the PHP version.
Note : Use version_compare() function and PHP_VERSION constant.
Sample Solution:
PHP Code:
<?php
// Check if PHP version is at least 6.0.0
if (version_compare(PHP_VERSION, '6.0.0') >= 0) {
echo 'I am at least PHP version 6.0.0, my version: ' . PHP_VERSION . "\n";
}
// Check if PHP version is at least 5.3.0
if (version_compare(PHP_VERSION, '5.3.0') >= 0) {
echo 'I am at least PHP version 5.3.0, my version: ' . PHP_VERSION . "\n";
}
// Check if PHP version is at least 5.0.0
if (version_compare(PHP_VERSION, '5.0.0', '>=')) {
echo 'I am using PHP 5, my version: ' . PHP_VERSION . "\n";
}
// Check if PHP version is less than 5.0.0
if (version_compare(PHP_VERSION, '5.0.0', '<')) {
echo 'I am using PHP 4, my version: ' . PHP_VERSION . "\n";
}
?>
Explanation:
- Check PHP Version 6.0.0 or Higher:
- The version_compare() function compares the current PHP version (PHP_VERSION) with '6.0.0'.
- If the current version is at least 6.0.0, it echoes a message stating that the version is 6.0.0 or higher.
- Check PHP Version 5.3.0 or Higher:
- A similar version_compare() check is performed for '5.3.0'.
- If the current version is at least 5.3.0, it echoes a message confirming this along with the current version.
- Check if Using PHP 5:
- The code checks if the current version is at least 5.0.0 using version_compare() and echoes a message indicating that PHP 5 is being used.
- Check for PHP Version Less than 5.0.0:
- Finally, the code checks if the current version is less than 5.0.0.
- If true, it echoes a message indicating that PHP 4 is being used.
- Output of Current Version:
- Each condition outputs the current PHP version using the predefined constant PHP_VERSION.
Output:
I am at least PHP version 6.0.0, my version: 7.0.15-0ubuntu0.16.04.4 I am at least PHP version 5.3.0, my version: 7.0.15-0ubuntu0.16.04.4 I am using PHP 5, my version: 7.0.15-0ubuntu0.16.04.4
Flowchart:
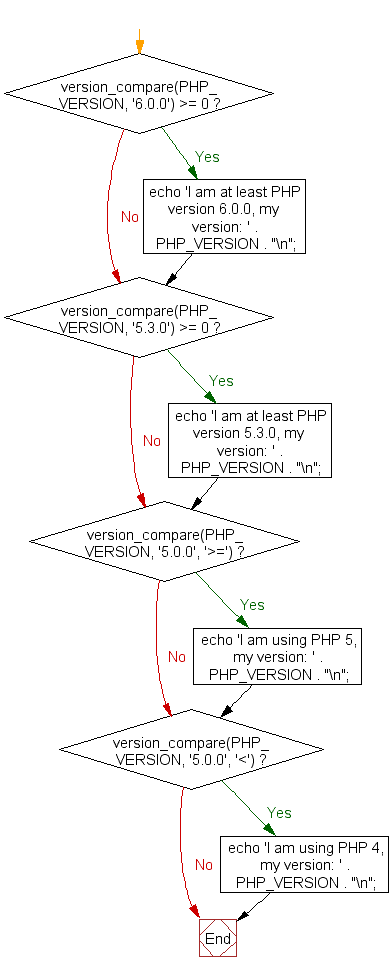
For more Practice: Solve these Related Problems:
- Write a PHP script to compare the current PHP version with a target version and output a compatibility report.
- Write a PHP script to check version compatibility for multiple PHP modules using version_compare().
- Write a PHP script to trigger an upgrade suggestion if the PHP version is below a predefined threshold.
- Write a PHP script to compare two version strings obtained from different sources using version_compare() and display the result.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a PHP script to get the full URL.
Next: Write a PHP script to get the name of the owner of the current PHP script.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.