PHP Exercises : Test whether a number is greater than 30, 20 or 10 using ternary operator
21. Ternary Operator Number Test
Write a PHP function to test whether a number is greater than 30, 20 or 10 using ternary operator.
Sample Solution: -
PHP Code:
<?php
// Function to test a given number using ternary operators
function trinary_Test($n){
// Ternary operators used to check the value of $n and assign a corresponding message to $r
$r = $n > 30
? "greater than 30"
: ($n > 20
? "greater than 20"
: ($n > 10
? "greater than 10"
: "Input a number at least greater than 10!"));
// Display the result with the input number
echo $n . " : " . $r . "\n";
}
// Test the function with different input values
trinary_Test(32);
trinary_Test(21);
trinary_Test(12);
trinary_Test(4);
?>
Explanation:
- Function Definition:
- The function trinary_Test($n) takes one parameter, $n, which represents the number to be tested.
- Ternary Operators:
- Inside the function, a series of nested ternary operators are used to evaluate the value of $n:
- If $n is greater than 30, it assigns the string "greater than 30" to $r.
- If $n is greater than 20, it assigns "greater than 20".
- If $n is greater than 10, it assigns "greater than 10".
- If none of the above conditions are met, it assigns "Input a number at least greater than 10!".
- Display Result:
- The function uses echo to output the input number and its corresponding message stored in $r, formatted as "<number> : <message>".
- Function Testing:
- The function is called four times with different input values: 32, 21, 12, and 4. Each call tests the number against the defined conditions and outputs the appropriate message.
Output:
32 : greater than 30 21 : greater than 20 12 : greater than 10 4 : Input a number atleast greater than 10!
Flowchart:
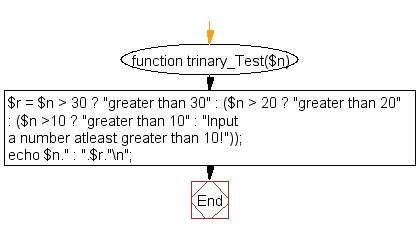
For more Practice: Solve these Related Problems:
- Write a PHP function using nested ternary operators to classify a number into multiple ranges.
- Write a PHP script to compare a number against several thresholds using a chained ternary operator.
- Write a PHP script to output different messages based on numeric ranges evaluated solely with ternary operators.
- Write a PHP function that accepts a number and returns a category label using nested ternary operators in a single expression.
Go to:
PREV : Get Last Error.
NEXT : Get Full URL.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.