PHP Exercises : Get the last occurred error
Write a PHP script to get the last occurred error.
Sample Solution:
PHP Code:
<?php
// Attempt to echo the variable $x (which is not defined)
echo $x;
// Print the details of the last error that occurred using error_get_last()
print_r(error_get_last());
?>
Explanation:
- Undefined Variable:
- echo $x tries to output the value of $x, but $x is not defined, which causes a PHP notice or warning error.
- Retrieve Last Error:
- error_get_last() returns an associative array with details of the last error that occurred, including the error type, message, file, and line number.
- Display Error Details:
- print_r(error_get_last()) outputs the array containing information about the last error (from the undefined variable), allowing for debugging or logging.
Output:
Array ( [type] => 8 [message] => Undefined variable: x [file] => /home/students/0d0149c0-f42e-11e6-a8c0-b738b9f f32f9.php [line] => 2 )
Flowchart:
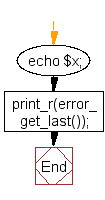
Note: error_get_last() function returns an associative array describing the last error with keys "type", "message", "file" and "line". If the error has been caused by a PHP internal function then the "message" begins with its name. Returns NULL if there hasn't been an error yet.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Arithmetic operations on character variables : $d = 'A00'. Using this variable print the following numbers.
Next: Write a PHP function to test whether a number is greater than 30, 20 or 10 using ternary operator.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics