PHP Exercises : Get last modified information of a file
Write a PHP script to get last modified information of a file.
Sample filename : php-basic-exercises.php
Sample Solution:
PHP Code:
<?php
// Get the current file name using basename and $_SERVER['PHP_SELF']
$current_file_name = basename($_SERVER['PHP_SELF']);
// Get the last modification time of the current file
$file_last_modified = filemtime($current_file_name);
// Display the last modified time in a human-readable format
echo "Last modified " . date("l, dS F, Y, h:ia", $file_last_modified) . "\n";
?>
Explanation:
- Get Current File Name:
- The basename() function, combined with $_SERVER['PHP_SELF'], retrieves the name of the currently executing PHP file and stores it in $current_file_name.
- Get Last Modification Time:
- filemtime($current_file_name) gets the last modification timestamp of the current file and stores it in $file_last_modified.
- Format Modification Time:
- The date() function converts $file_last_modified to a human-readable format ("l, dS F, Y, h:ia"), showing the day of the week, day, month, year, and time with AM/PM.
- Display Last Modified Time:
- The echo statement outputs the formatted last modified date and time (e.g., "Last modified Monday, 25th October, 2024, 03:15pm").
Output:
Last modified Monday, 26th June, 2017, 02:06pm
Flowchart:
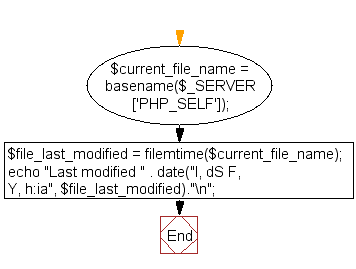
Note: The result may vary for your system date and time.
basename() function: The basename(path,suffix) function is used to get the filename from a path.
filemtime() function: The filemtime(filename) function is used to get the last time the file content was modified.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a PHP script to display source code of a webpage (e.g. "http://www.example.com/").
Next: Write a PHP script to count number of lines in a file.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics