PHP Exercises : Email validation
12. Email Validation
Write a simple PHP program to check that emails are valid.
Hints : Use FILTER_VALIDATE_EMAIL filter that validates value as an e-mail address.
Note : The PHP documentation does not say that FILTER_VALIDATE_EMAIL should pass the RFC5321.
Sample Solution:
PHP Code:
<?php
// Specify an email address (valid or invalid)
$email = "[email protected]";
// Use the filter_var function with FILTER_VALIDATE_EMAIL to check if the email is valid
if (filter_var($email, FILTER_VALIDATE_EMAIL))
{
// If the email is valid, print a message indicating it is valid
echo '"' . $email . '" = Valid'."\n";
}
else
{
// If the email is invalid, print a message indicating it is invalid
echo '"' . $email . '" = Invalid'."\n";
}
?>
Explanation:
- Specify Email Address:
- The $email variable is set to "[email protected]", which represents the email address to validate.
- Filter Validation:
- The filter_var() function is used with the FILTER_VALIDATE_EMAIL filter to check if the $email variable contains a valid email format.
- Conditional Check:
- An if statement checks the result of filter_var().
- If filter_var() returns true (indicating a valid email), the code inside the if block runs.
- Output for Valid Email:
- If the email is valid, it prints "<email> = Valid" to indicate that the email format is correct.
- Output for Invalid Email:
- If the email is not valid, it goes to the else block, printing "<email> = Invalid" to indicate an incorrect format.
- End of Script:
- The code ends after displaying whether the email is valid or invalid.
Output:
"[email protected]" = Valid
filter_var() function:
Syntax:
mixed filter_var ( mixed $variable [, int $filter = FILTER_DEFAULT [, mixed $options ]] )
filter_var() function filters a variable with a specified filter.
Parameters:
- variable: Value to filter.
- filter: The ID of the filter to apply.
- options: Associative array of options or bitwise disjunction of flags.
Flowchart:
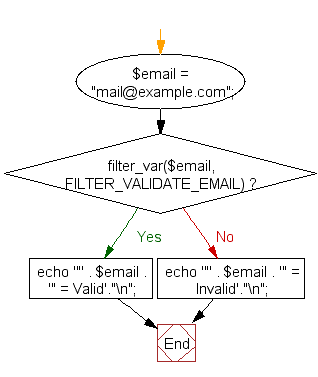
For more Practice: Solve these Related Problems:
- Write a PHP script to validate multiple email addresses using both FILTER_VALIDATE_EMAIL and regular expressions.
- Write a PHP script to validate an email address and perform a DNS lookup to verify the domain exists.
- Write a PHP script to sanitize user-supplied emails and output them in a standardized format.
- Write a PHP script to accept an array of emails and return a list of those that fail validation.
Go to:
PREV : Page Redirection.
NEXT : Display Values in Table.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.