PHP Exercises: Capture a variable number of arguments to a given function
PHP: Exercise-102 with Solution
Write a PHP program to capture a variable number of arguments to a given function.
Sample Solution:
PHP Code:
<?php
// Licence: https://bit.ly/2CFA5XY
// Function definition for 'variadicFunction' that takes an array of operands as a parameter
function variadicFunction($operands)
{
// Initialize a variable to store the sum of operands
$sum = 0;
// Iterate through each operand in the array and add it to the sum
foreach($operands as $singleOperand) {
$sum += $singleOperand;
}
// Return the final sum
return $sum;
}
// Call 'variadicFunction' with an array containing two operands and display the result using 'var_dump'
var_dump(variadicFunction([1, 2]));
// Call 'variadicFunction' with an array containing four operands and display the result using 'var_dump'
var_dump(variadicFunction([1, 2, 3, 4]));
?>
Sample Output:
int(3) int(10)
Flowchart:
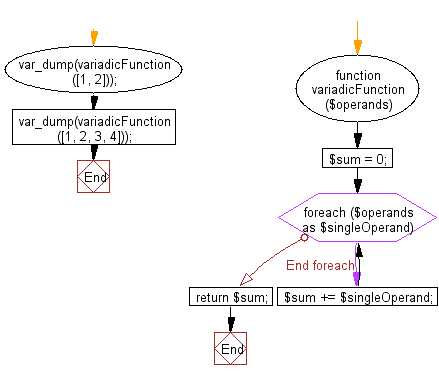
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous:Write a PHP program to curry a function to take arguments in multiple calls.
Next: PHP Array Exercises Home.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics