PHP Exercises: Call a given function only once
101. Call a Function Only Once
Write a PHP program to call a given function only once.
Sample Solution:
PHP Code:
<?php
// Licence: https://bit.ly/2CFA5XY
// Function definition for 'once' that takes a function as a parameter
function once($function)
{
// Return a closure that encapsulates the original function
return function (...$args) use ($function) {
// Static variable to track whether the function has been called
static $called = false;
// If the function has been called before, return early without executing the original function
if ($called) {
return;
}
// Mark the function as called and execute the original function with the provided arguments
$called = true;
return $function(...$args);
};
}
// Anonymous function definition for 'add' that takes two parameters and returns their sum
$add = function ($a, $b) {
return $a + $b;
};
// Create a 'once' version of the 'add' function
$once = once($add);
// Call the 'once' version of 'add' with arguments and display the result using 'var_dump'
var_dump($once(10, 5)); // Output: int(15)
// Attempt to call the 'once' version of 'add' again with different arguments
// The second call should return early without executing the original function
var_dump($once(20, 10)); // Output: NULL
?>
Explanation:
- Function Definition:
- The once function is defined to accept another function as its parameter.
- Closure Creation:
- Inside once, a closure is returned that captures the original function using the use keyword.
- Static Variable:
- A static variable $called is initialized to false to track whether the original function has been executed.
- First Call Check:
- The closure checks if $called is true. If it is, the function returns early without executing the original function.
- Marking the Function as Called:
- If the function has not been called before, $called is set to true, and the original function is executed with the provided arguments.
- Anonymous Function for Addition:
- An anonymous function $add is defined to take two parameters and return their sum.
- Once-ified Function:
- The once function is called with the $add function, creating a version of $add that can only be executed once.
- First Function Call:
- The once version of the add function is called with arguments 10 and 5. The result (15) is displayed using var_dump.
- Second Function Call:
- The once version of the add function is called again with different arguments (20 and 10).
- This call does not execute the original function, as it has already been called once, resulting in NULL being displayed.
Output:
int(15) NULL
Flowchart:
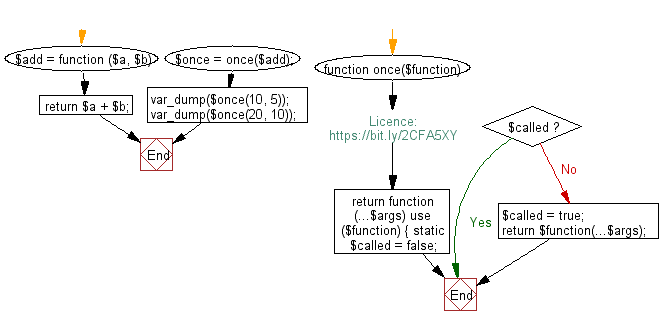
For more Practice: Solve these Related Problems:
- Write a PHP script to wrap a function so that it executes only on the first call and ignores subsequent invocations.
- Write a PHP function to cache the result of its initial execution and return NULL for later calls.
- Write a PHP script to implement a "call once" mechanism using static variables to track invocation.
- Write a PHP script to ensure that a specific function is executed only one time regardless of repeated calls.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous:Write a PHP program to curry a function to take arguments in multiple calls.
Next: Write a PHP program to capture a variable number of arguments to a given function.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.