PHP Array Exercises : Calculate and display average temperature
PHP Array: Exercise-9 with Solution
Write a PHP script to calculate and display average temperature, five lowest and highest temperatures.
Recorded temperatures : 78, 60, 62, 68, 71, 68, 73, 85, 66, 64, 76, 63, 75, 76, 73, 68, 62, 73, 72, 65, 74, 62, 62, 65, 64, 68, 73, 75, 79, 73
Sample Solution:
PHP Code:
<?php
// Define a string of monthly temperatures separated by commas
$month_temp = "78, 60, 62, 68, 71, 68, 73, 85, 66, 64, 76, 63, 81, 76, 73,
68, 72, 73, 75, 65, 74, 63, 67, 65, 64, 68, 73, 75, 79, 73";
// Split the string into an array using commas as the delimiter
$temp_array = explode(',', $month_temp);
// Initialize variables for total temperature and the length of the temperature array
$tot_temp = 0;
$temp_array_length = count($temp_array);
// Iterate through the temperature array, calculate the total temperature
foreach($temp_array as $temp)
{
$tot_temp += $temp;
}
// Calculate the average high temperature
$avg_high_temp = $tot_temp / $temp_array_length;
// Display the average temperature
echo "Average Temperature is : " . $avg_high_temp . "";
// Sort the temperature array in ascending order
sort($temp_array);
// Display the list of five lowest temperatures
echo " List of five lowest temperatures :";
for ($i = 0; $i < 5; $i++)
{
echo $temp_array[$i] . ", ";
}
// Display the list of five highest temperatures
echo "List of five highest temperatures :";
for ($i = ($temp_array_length - 5); $i < $temp_array_length; $i++)
{
echo $temp_array[$i] . ", ";
}
?>
Output:
Average Temperature is : 70.6 List of five lowest temperatures : 60, 62, 63, 63, 64, List of five highest temperatures : 76, 78, 79, 81, 85,
Flowchart:
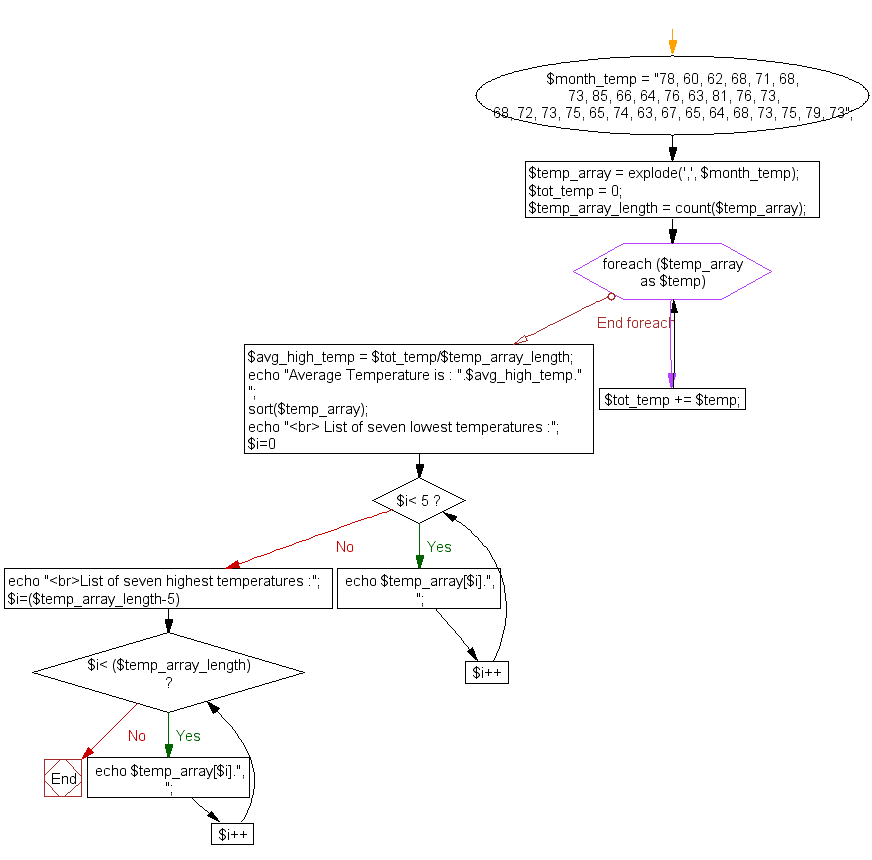
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP script to sort the specified associative array.
Next: Write a PHP program to sort an array of positive integers using the Bead-Sort Algorithm.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics