PHP Array Exercises : Inserts a new item in an array in any position
Write a PHP script that inserts a new item in an array in any position.
Sample Solution:
PHP Code:
<?php
// Define an indexed array $original with elements '1', '2', '3', '4', '5'
$original = array( '1', '2', '3', '4', '5' );
// Output a message indicating the original array
echo 'Original array : ' . "\n";
// Iterate through the elements of the original array and echo them
foreach ($original as $x) {
echo "$x ";
}
// Define a string '$' to be inserted into the array
$inserted = '$';
// Use array_splice() to insert the value '$' at index 3 in the original array
array_splice($original, 3, 0, $inserted);
// Output a message indicating the array after the insertion
echo " \n After inserting '$' the array is : " . "\n";
// Iterate through the modified array and echo its elements
foreach ($original as $x) {
echo "$x ";
}
// Output a newline character for better formatting
echo "\n";
?>
Output:
Original array : 1 2 3 4 5 After inserting '$' the array is : 1 2 3 $ 4 5
Flowchart:
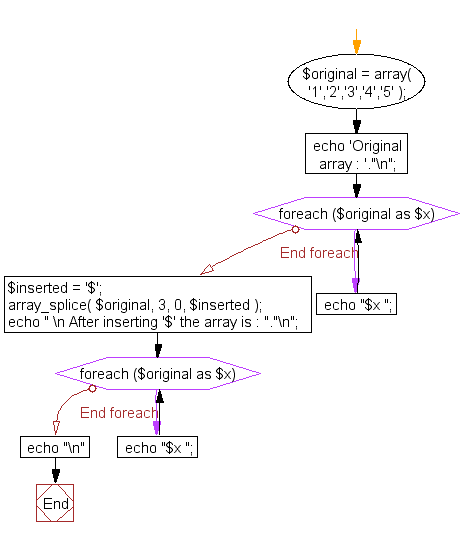
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP script which decodes the specified JSON string.
Next: Write a PHP script to sort the specified associative array
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics