PHP Array Exercises : Decodes a JSON string
6. Decode JSON String
Write a PHP script which decodes the following JSON string.
Sample JSON code :
{"Title": "The Cuckoos Calling",
"Author": "Robert Galbraith",
"Detail": {
"Publisher": "Little Brown"
}}
Sample Solution:
PHP Code:
<?php
// Define a function named w3rfunction that echoes the key and value of an array element
function w3rfunction($value, $key) {
echo "$key : $value" . "\n";
}
// Define a JSON-encoded string representing a nested associative array
$a = '{"Title": "The Cuckoos Calling",
"Author": "Robert Galbraith",
"Detail": {
"Publisher": "Little Brown"
}
}';
// Decode the JSON string into a PHP associative array
$j1 = json_decode($a, true);
// Use array_walk_recursive to apply the w3rfunction to each element in the nested array
array_walk_recursive($j1, "w3rfunction");
?>
Output:
Title : The Cuckoos Calling Author : Robert Galbraith Publisher : Little Brown
Flowchart:
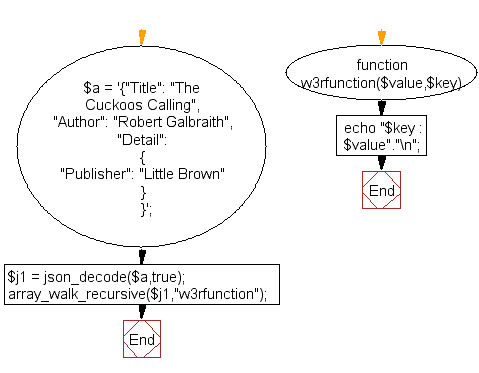
For more Practice: Solve these Related Problems:
- Write a PHP script to decode a JSON string containing nested objects and then output specific properties formatted as plain text.
- Write a PHP function that takes a JSON string, decodes it into an associative array, and prints each key-value pair on a new line.
- Write a PHP program to decode a JSON string, validate its structure, and then iterate over its elements to display content in a tabular format.
- Write a PHP script to handle errors during JSON decoding, outputting an appropriate message if the JSON is invalid.
Go to:
PREV : Get First Element from Associative Array.
NEXT : Insert New Array Item by Position.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.