PHP Array Exercises : Combine two arrays
58. Combine Two Arrays (Keys and Values)
Write a PHP script to combine (using one array for keys and another for its values) the following two arrays.
('x', 'y', 'y'), (10, 20, 30)
Sample Solution:
PHP Code:
<?php
// Function to combine two arrays into an associative array
function combine_Array($keys, $values)
{
// Initialize an empty array to store the combined result
$result = array();
// Iterate through each element of the $keys array
foreach ($keys as $i => $k) {
// Use the keys as indices and group values accordingly
$result[$k][] = $values[$i];
}
// Use array_walk to iterate through each element in $result and modify it
array_walk($result, function (&$v) {
// If an array has only one element, replace it with that element
$v = (count($v) == 1) ? array_pop($v) : $v;
});
// Return the combined array
return $result;
}
// Two arrays to be combined
$array1 = array('x', 'y', 'y');
$array2 = array(10, 20, 30);
// Print the result of combining the arrays
print_r(combine_Array($array1, $array2));
?>
Output:
Array ( [x] => 10 [y] => Array ( [0] => 20 [1] => 30 ) )
Flowchart:
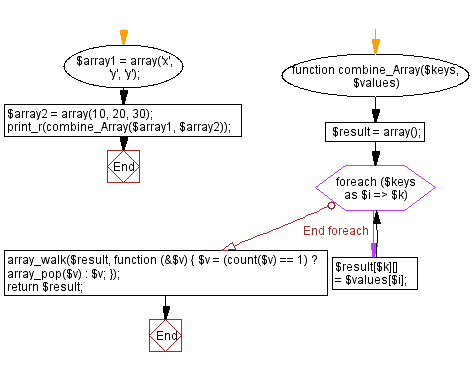
For more Practice: Solve these Related Problems:
- Write a PHP script to merge two arrays where one array provides keys and the other provides values, then output the resulting associative array.
- Write a PHP function to combine an array of keys and an array of values into a single associative array, handling mismatched lengths.
- Write a PHP program to use array_combine() to merge two given arrays and then validate the output structure.
- Write a PHP script to simulate the combination of two arrays without using array_combine(), by manually iterating over the elements.
Go to:
PREV : Compare Two Multidimensional Arrays for Difference.
NEXT : Create a Range-Like Array from a String.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.