PHP Array Exercises : Get the last value of an array without affecting the pointer
50. Get Last Array Value Without Affecting Pointer
Write a PHP script to get the last value of an array without affecting the pointer.
Sample Solution:
PHP Code:
<?php
// Original associative array
$colors = array('c1' => 'Red', 'c2' => 'Green', 'c3' => 'White', 'c4' => 'Black');
// Move the internal pointer to the next element and return its value
echo next($colors) . "\n";
// Use array_flip to swap keys and values, then array_keys to get the keys
$keys = array_keys(array_flip($colors));
// Use array_pop to get the last element
$last = array_pop($keys);
echo $last . "\n";
// Move the internal pointer to the first element and return its value
echo current($colors) . "\n";
?>
Output:
Green Black Green
Flowchart:
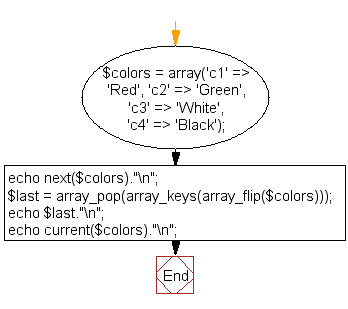
For more Practice: Solve these Related Problems:
- Write a PHP script to retrieve the last element of an array without modifying the internal pointer by using end() while preserving the pointer's original position.
- Write a PHP function to get the last value of an array and then reset the array pointer to its original state.
- Write a PHP program to implement a custom function to return the last element without changing the pointer using array_slice().
- Write a PHP script to output the last element of an array while simultaneously displaying the pointer's value before and after the operation.
Go to:
PREV : Get Array Entries with Specific Keys.
NEXT : Filter Out Elements with Certain Key-Names.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.