PHP Array Exercises : Get an array with the first key and value
47. Get an Array with First Key and Value
Write a PHP function to get an array with the first key and value.
Sample Solution:
PHP Code:
<?php
// Function to extract and return the first element of an associative array
function array_1st_element($my_array)
{
// Extract the first key of the array using list() and array_keys()
list($k) = array_keys($my_array);
// Create a new array with the first key and its corresponding value
$result = array($k=>$my_array[$k]);
// Remove the first element from the original array
unset($my_array[$k]);
// Return the result array containing the first element
return $result;
}
// Test array
$colors = array('c1'=>'Red','c2'=>'Green','c3'=>'Black');
// Call the function and display the result
print_r(array_1st_element($colors));
?>
Output:
Array ( [c1] => Red )
Flowchart:
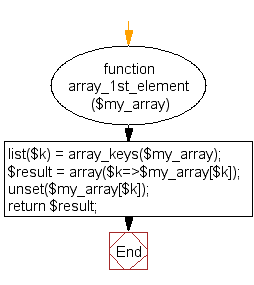
For more Practice: Solve these Related Problems:
- Write a PHP function to extract the first key-value pair from an associative array and return it as a new array.
- Write a PHP script to use key() and current() functions to create an array containing only the first element.
- Write a PHP program to reset an array pointer and then store the first key and value in a new, single-element associative array.
- Write a PHP script to loop over an associative array and break after the first iteration, capturing the key and value.
Go to:
PREV : Check if All Array Values Are Strings.
NEXT : Set Union of Two Arrays.
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.